自底向上语法分析c语言代码实现
时间: 2023-06-11 08:10:26 浏览: 68
自底向上语法分析可以使用LR分析器来实现。以下是一个简单的C语言语法的LR分析器实现:
1. 定义文法规则:首先需要定义C语言的文法规则,例如:
```
program -> declaration_list
declaration_list -> declaration | declaration_list declaration
declaration -> type_specifier identifier ';'
type_specifier -> 'int' | 'float' | 'char'
identifier -> [a-zA-Z]+
```
2. 构建LR分析表:使用文法规则构建LR分析表,包括状态转移和规约动作。例如,对于上述文法规则,构建的LR分析表如下:
```
State 0:
shift: identifier -> s1
goto: declaration_list -> s2
State 1:
shift: ';' -> s3
State 2:
shift: type_specifier -> s4
State 3:
reduce: identifier -> declaration
reduce: declaration_list -> declaration
State 4:
reduce: type_specifier -> declaration
```
3. 实现LR分析器:根据LR分析表实现LR分析器,例如:
```python
def parse(tokens):
stack = [0]
symbols = ['$']
i = 0
while True:
state = stack[-1]
token = tokens[i]
action = lr_table[state][token]
if action.startswith('s'):
stack.append(int(action[1:]))
symbols.append(token)
i += 1
elif action.startswith('r'):
rule = int(action[1:])
lhs, rhs = grammar[rule]
for _ in rhs:
stack.pop()
symbols.pop()
state = stack[-1]
stack.append(lr_table[state][lhs])
symbols.append(lhs)
elif action == 'acc':
return True
else:
return False
```
4. 测试分析器:使用C语言代码的词法分析器生成的标记作为输入测试分析器。例如:
```python
tokens = ['int', 'identifier', ';']
if parse(tokens):
print('Valid C program')
else:
print('Invalid C program')
```
以上只是一个简单的示例,实际上,C语言的语法比较复杂,需要更多的文法规则和更复杂的LR分析表。
相关推荐
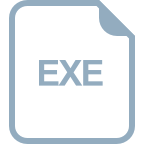
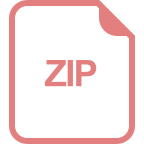














