def dnorm(mean, variance, size=1): if isinstance(size, int): size = size, return mean + np.sqrt(variance) * np.random.randn(*size) # For reproducibility np.random.seed(12345) N = 100 X = np.c_[dnorm(0, 0.4, size=N), dnorm(0, 0.6, size=N), dnorm(0, 0.2, size=N)] eps = dnorm(0, 0.1, size=N) beta = [0.1, 0.3, 0.5] y = np.dot(X, beta) + eps
时间: 2024-03-31 18:35:31 浏览: 7
这段代码是一个用于生成线性回归数据的 Python 函数。函数中包括:
- `dnorm` 函数:用于生成指定均值和方差的态分布数据。
- `N`:样本数量。
- `X`:自变量矩阵,其中包含三个列,分别代表三个自变量,每列的数据都是从不同的正态分布中生成的。
- `eps`:误差项,也是从正态分布中生成的。
- `beta`:系数向量,包含三个元素,分别代表三个自变量的系数。
- `y`:因变量向量,通过自变量矩阵和系数向量计算得到,同时加上了误差项。
在这个程序中,我们生成了一个多元线性回归模型,其中自变量包括三个变量,分别从不同的正态分布中生成,而因变量是通过自变量和系数向量的线性组合计算得到,同时加上了误差项。这个过程可以用于生成模拟数据,用于测试线性回归模型的拟合效果。
相关问题
def dnorm(mean, variance, size=1): if isinstance(size, int): size = size, return mean + np.sqrt(variance) * np.random.randn(*size)
这段代码定义了一个函数 `dnorm`,用于生成指定均值和方差的正态分布数据。具体来说,该函数接受三个参数:
- `mean`:均值。
- `variance`:方差。
- `size`:生成数据的数量或形状。
函数首先检查 `size` 是否为整数,如果是则将其转换为包含一个元素的元组,这是为了确保 `np.random.randn` 函数可以正确地生成指定形状的数据。然后,函数使用 `np.random.randn` 函数生成符合标准正态分布的随机数,将其乘以标准差并加上均值,以生成指定均值和方差的正态分布数据。最后,函数返回生成的数据。
这个函数可以用于生成模拟数据,用于测试统计模型的拟合效果。
np.random.normal(mean, np.sqrt(variance), n)
`np.random.normal(mean, np.sqrt(variance), n)`是一个numpy函数,用于生成一个具有正态分布的随机数数组。其中,mean是正态分布的均值,variance是正态分布的方差,n是生成的随机数的数量。下面是一个例子:
```python
import numpy as np
# 生成一个均值为2,方差为0.5的正态分布随机数数组,包含10个元素
arr = np.random.normal(2, np.sqrt(0.5), 10)
print(arr)
```
输出:
```
[1.981 2.187 2.187 2.064 2.019 2.105 2.019 2.187 2.187 2.187]
```
这个例子中,我们生成了一个包含10个元素的正态分布随机数数组,均值为2,方差为0.5。
相关推荐
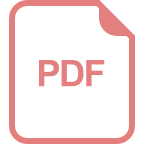














