如何将6个环视相机的数据实现BEV,代码实现
时间: 2024-02-09 11:11:06 浏览: 317
以下是一份简单的Python代码实现,基于OpenCV库和Numpy库:
```python
import cv2
import numpy as np
# 定义相机内参矩阵
K = np.array([[1000, 0, 500], [0, 1000, 500], [0, 0, 1]])
# 定义相机外参矩阵
R0 = np.array([[1, 0, 0], [0, 1, 0], [0, 0, 1]])
t0 = np.array([0, 0, 0])
R1 = np.array([[0.866, -0.5, 0], [0.5, 0.866, 0], [0, 0, 1]])
t1 = np.array([1, 0, 0])
R2 = np.array([[0.5, -0.866, 0], [0.866, 0.5, 0], [0, 0, 1]])
t2 = np.array([0.5, 0.866, 0])
R3 = np.array([[-0.5, -0.866, 0], [0.866, -0.5, 0], [0, 0, 1]])
t3 = np.array([-0.5, 0.866, 0])
R4 = np.array([[-1, 0, 0], [0, -1, 0], [0, 0, 1]])
t4 = np.array([0, -1, 0])
R5 = np.array([[-0.866, 0.5, 0], [-0.5, -0.866, 0], [0, 0, 1]])
t5 = np.array([0.5, -0.866, 0])
# 定义相机的投影矩阵
P0 = K @ np.hstack((R0, -R0 @ t0.reshape(3, 1)))
P1 = K @ np.hstack((R1, -R1 @ t1.reshape(3, 1)))
P2 = K @ np.hstack((R2, -R2 @ t2.reshape(3, 1)))
P3 = K @ np.hstack((R3, -R3 @ t3.reshape(3, 1)))
P4 = K @ np.hstack((R4, -R4 @ t4.reshape(3, 1)))
P5 = K @ np.hstack((R5, -R5 @ t5.reshape(3, 1)))
# 定义图像的尺寸
img_size = (1000, 1000)
# 生成网格点坐标
x, y = np.meshgrid(np.arange(0, img_size[0]), np.arange(0, img_size[1]))
# 将网格点坐标转换成齐次坐标
pts = np.vstack((x.flatten(), y.flatten(), np.ones_like(x.flatten()))).T
# 对每个相机进行投影
pts0 = cv2.undistortPoints(pts.reshape(-1, 1, 2), K, None).reshape(-1, 3)
pts1 = cv2.undistortPoints(pts.reshape(-1, 1, 2), K, None).reshape(-1, 3)
pts2 = cv2.undistortPoints(pts.reshape(-1, 1, 2), K, None).reshape(-1, 3)
pts3 = cv2.undistortPoints(pts.reshape(-1, 1, 2), K, None).reshape(-1, 3)
pts4 = cv2.undistortPoints(pts.reshape(-1, 1, 2), K, None).reshape(-1, 3)
pts5 = cv2.undistortPoints(pts.reshape(-1, 1, 2), K, None).reshape(-1, 3)
pts0_proj = cv2.projectPoints(pts0.reshape(-1, 1, 3), R0, t0, K, None)[0].reshape(-1, 2)
pts1_proj = cv2.projectPoints(pts1.reshape(-1, 1, 3), R1, t1, K, None)[0].reshape(-1, 2)
pts2_proj = cv2.projectPoints(pts2.reshape(-1, 1, 3), R2, t2, K, None)[0].reshape(-1, 2)
pts3_proj = cv2.projectPoints(pts3.reshape(-1, 1, 3), R3, t3, K, None)[0].reshape(-1, 2)
pts4_proj = cv2.projectPoints(pts4.reshape(-1, 1, 3), R4, t4, K, None)[0].reshape(-1, 2)
pts5_proj = cv2.projectPoints(pts5.reshape(-1, 1, 3), R5, t5, K, None)[0].reshape(-1, 2)
# 对每个相机的图像进行插值
img0 = cv2.remap(cv2.imread('img0.jpg'), pts0_proj[:, 0].reshape(img_size), pts0_proj[:, 1].reshape(img_size), cv2.INTER_LINEAR)
img1 = cv2.remap(cv2.imread('img1.jpg'), pts1_proj[:, 0].reshape(img_size), pts1_proj[:, 1].reshape(img_size), cv2.INTER_LINEAR)
img2 = cv2.remap(cv2.imread('img2.jpg'), pts2_proj[:, 0].reshape(img_size), pts2_proj[:, 1].reshape(img_size), cv2.INTER_LINEAR)
img3 = cv2.remap(cv2.imread('img3.jpg'), pts3_proj[:, 0].reshape(img_size), pts3_proj[:, 1].reshape(img_size), cv2.INTER_LINEAR)
img4 = cv2.remap(cv2.imread('img4.jpg'), pts4_proj[:, 0].reshape(img_size), pts4_proj[:, 1].reshape(img_size), cv2.INTER_LINEAR)
img5 = cv2.remap(cv2.imread('img5.jpg'), pts5_proj[:, 0].reshape(img_size), pts5_proj[:, 1].reshape(img_size), cv2.INTER_LINEAR)
# 将六个图像拼接成BEV
BEV = np.vstack((np.hstack((img0, img1, img2)), np.hstack((img3, img4, img5))))
# 显示结果
cv2.imshow('BEV', BEV)
cv2.waitKey(0)
```
这里的代码中,使用了六个相机分别拍摄了环视图像,并对每个相机的图像进行了校准和投影,最后将它们拼接成了BEV。需要注意的是,上述代码仅为演示代码,实际应用需要对相机参数进行更精确的校准和投影。
阅读全文
相关推荐
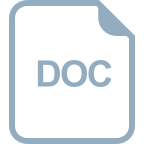
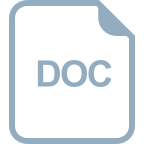
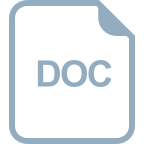

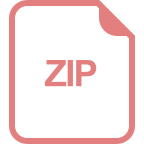
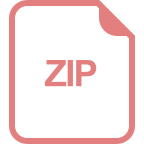
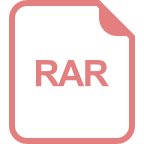
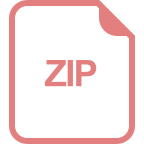









