A positive integer n is said to be perfect if it is equal to the sum of its proper factors (i.e. factors other than itself). So for instance, the number 28 is perfect: its proper factors are 1,2,4,7,14, and 1+2+4+7+14=28. On the other hand, 20 isn't perfect, as its factors are 1,2,4,5,10 and 1+2+4+5+10=22>20. Write a method isPerfect(int toCheck) that takes one parameter of type int, and returns true if toCheck is a perfect number, false otherwise. Note: you may assume that the integer toCheck is positive.
时间: 2023-03-29 22:03:56 浏览: 174
我可以回答这个问题。以下是一个判断一个正整数是否为完美数的方法:
```
public static boolean isPerfect(int toCheck) {
int sum = ;
for (int i = 1; i < toCheck; i++) {
if (toCheck % i == ) {
sum += i;
}
}
return sum == toCheck;
}
```
该方法首先定义一个变量 sum,用于存储 toCheck 的所有因子之和。然后使用一个 for 循环,从 1 到 toCheck - 1 遍历所有可能的因子。如果 i 是 toCheck 的因子,则将其加入到 sum 中。最后,如果 sum 等于 toCheck,则 toCheck 是完美数,返回 true;否则返回 false。
相关问题
Given a positive integer 'n', the task is to find a prime number greater than or equal to 'n'. the output should be y
Sure, I can help you with that. Here's a Python code snippet that finds the next prime number greater than or equal to a given integer 'n':
```python
def is_prime(num):
"""
Returns True if the given number is prime, False otherwise.
"""
if num < 2:
return False
for i in range(2, int(num ** 0.5) + 1):
if num % i == 0:
return False
return True
def next_prime(n):
"""
Returns the next prime number greater than or equal to the given number.
"""
num = n
while True:
if is_prime(num):
return num
num += 1
# Example usage
n = 10
print(next_prime(n)) # Output: 11
```
The `is_prime()` function checks if a given number is prime or not by iterating from 2 to the square root of the number and checking if it is divisible by any of the numbers in between.
The `next_prime()` function takes an integer `n` as input and starts iterating from `n` until it finds the next prime number using the `is_prime()` function. Once it finds a prime number, it returns it as output.
Hope this helps!
Given an array of positive integers nums and a positive integer target, return the minimal length of a subarray whose sum is greater than or equal to target. If there is no such subarray, return 0 instead.
This problem can be solved using the sliding window technique. We maintain a window of elements whose sum is greater than or equal to the target. We start with two pointers, left and right, both pointing to the first element of the array. We then move the right pointer to the right until the sum of elements in the window is greater than or equal to the target. Once we have found such a window, we update the answer with the length of the current window. We then move the left pointer to the right until the sum of elements in the window is less than the target. We repeat this process until the right pointer reaches the end of the array. We return the answer.
Here is the Python code:
```
def minSubArrayLen(nums, target):
n = len(nums)
left = right = 0
window_sum = 0
ans = float('inf')
while right < n:
window_sum += nums[right]
while window_sum >= target:
ans = min(ans, right - left + 1)
window_sum -= nums[left]
left += 1
right += 1
return ans if ans != float('inf') else 0
```
The time complexity of this algorithm is O(n) and the space complexity is O(1).
阅读全文
相关推荐
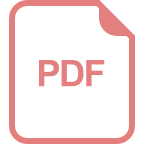
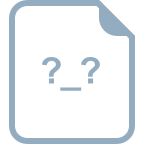
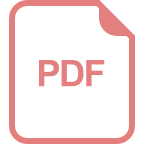










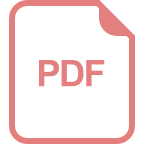
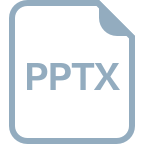
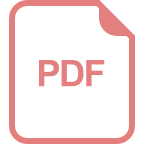
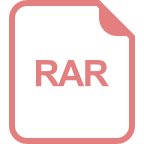