Given a positive integer 'n', the task is to find a prime number greater than or equal to 'n'. Example 1 If given 'n' is 10, then the closest prime number greater than 10 is 11 at a distance of 1. So 11 must be returned. Example 2 If the given 'n' is 17, then the closest prime number is itself at a distance of 0. So 17 must be returned. The returned prime number must be greater than or equal to 'n'.
时间: 2023-12-03 07:44:18 浏览: 28
Here's a Python code that finds the closest prime number greater than or equal to n:
```python
def is_prime(num):
"""
Returns True if the given number is prime, False otherwise.
"""
if num <= 1:
return False
for i in range(2, int(num ** 0.5) + 1):
if num % i == 0:
return False
return True
def find_closest_prime(n):
"""
Finds the closest prime number greater than or equal to n.
"""
if is_prime(n):
return n
else:
i = n
while True:
if is_prime(i):
return i
i += 1
```
The function `is_prime` checks if a given number is prime by checking if it is divisible by any number between 2 and the square root of the number.
The function `find_closest_prime` first checks if n is already a prime number. If so, it returns n. Otherwise, it starts checking the next numbers (i.e., i = n+1, n+2, n+3, ...) until it finds a prime number, which it then returns.
相关推荐
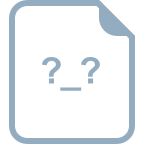
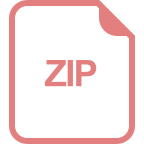















