帮我用python写一个prim算法
时间: 2023-05-16 10:06:33 浏览: 52
当然可以,以下是一个基本的Prim算法的Python实现:
```python
from queue import PriorityQueue
def prim(graph, start):
visited = set()
pq = PriorityQueue()
mst = []
visited.add(start)
for neighbor, weight in graph[start].items():
pq.put((weight, start, neighbor))
while not pq.empty():
weight, u, v = pq.get()
if v not in visited:
visited.add(v)
mst.append((u, v, weight))
for neighbor, weight in graph[v].items():
pq.put((weight, v, neighbor))
return mst
```
其中,`graph`是一个字典,表示图的邻接表,`start`是起点。返回值是一个列表,表示最小生成树的边集。
注意,这只是一个基本的实现,可能存在一些性能问题和边界情况没有考虑到。如果需要更加健壮的实现,可以参考其他资料或者自行优化。
相关问题
用python写一个prim算法
Prim算法是一种用于计算最小生成树的算法,它通过不断找到最小边来不断扩展生成树,从而找到整张图的最小生成树。下面是一种用Python实现Prim算法的方法:
1. 初始化:将图的某一个顶点作为起点,并将其加入生成树中。
2. 找到最小边:在剩下的顶点中找到一条连接已加入生成树的顶点和未加入生成树的顶点的边,并将该顶点加入生成树中。
3. 重复步骤2,直到所有的顶点都加入生成树为止。
下面是一个简单的Prim算法的Python代码实现:
```
import sys
class Prim:
def __init__(self, graph):
self.graph = graph
self.V = len(graph)
self.mst = [False] * self.V
self.key = [sys.maxsize] * self.V
self.parent = [None] * self.V
def primMST(self):
self.key[0] = 0
self.parent[0] = -1
for i in range(self.V):
u = self.minKey()
self.mst[u] = True
for v in range(self.V):
if self.graph[u][v] > 0 and self.mst[v] == False and self.key[v] > self.graph[u][v]:
self.key[v] = self.graph[u][v]
self.parent[v] = u
def minKey(self):
min = sys.maxsize
min_index = 0
for v in range(self.V):
if self.mst[v] == False and self.key[v] < min:
min = self.key[v]
min_index = v
return min_index
def printMST(self):
for i in range(1, self.V):
print("%d - %d: %d" % (self.parent[i], i, self.graph[i][self.parent[i]]))
```
用
用python写一个求最小生成树prim算法代码
当然可以,以下是基于 Python 的 Prim 算法代码实现。
```python
import heapq
def prim(graph):
"""
Prim's algorithm to find minimum spanning tree of a graph.
"""
visited = set()
edges = [(0, None, graph.keys()[0])]
mst = []
while edges:
weight, prev, node = heapq.heappop(edges)
if node not in visited:
visited.add(node)
mst.append((prev, node, weight))
for neighbor, w in graph[node].items():
if neighbor not in visited:
heapq.heappush(edges, (w, node, neighbor))
return mst
```
其中,使用了 Python 自带的 `heapq` 模块来实现最小堆,优化了 Prim 算法的时间复杂度。
输入的 `graph` 参数是一个字典,其中键是节点,值是另一个字典,表示邻居和它们之间的权重。这可以通过以下方式创建:
```python
graph = {
'A': {'B': 2, 'C': 3, 'D': 1},
'B': {'A': 2, 'E': 3},
'C': {'A': 3, 'E': 1},
'D': {'A': 1},
'E': {'B': 3, 'C': 1}
}
```
最后,返回一个列表,其中每个元素都是一个三元组,表示连接两个顶点的边和它们之间的权重。
相关推荐
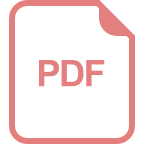












