在串行实现π计算基础上,按照任务分解思想,对问题进行并行化改造,并实现代码。 实现语言不限。 根据任务分解思想要求, 1、使用单线程计算π、两线程计算π、四线程计算π 2、在程序中,必须记下不同线程计算π的时间 3、输出格式: 单线程时间:2.000秒 2线程时间:1.5000秒 4线程时间:1.0000秒
时间: 2023-03-24 11:03:51 浏览: 49
好的,我可以回答这个问题。根据任务分解思想,我们可以将π的计算任务分解成多个子任务,然后分配给不同的线程并行计算。具体实现可以使用多线程编程技术,例如使用 pthread 库实现。以下是示例代码:
```
#include <stdio.h>
#include <stdlib.h>
#include <pthread.h>
#include <sys/time.h>
#define NUM_THREADS 4
double pi = .;
int num_steps = 100000000;
void *calc_pi(void *threadid) {
long tid = (long)threadid;
int start = tid * num_steps / NUM_THREADS;
int end = (tid + 1) * num_steps / NUM_THREADS;
double sum = .;
double x;
for (int i = start; i < end; i++) {
x = (i + .5) / num_steps;
sum += 4. / (1. + x * x);
}
pi += sum / num_steps;
pthread_exit(NULL);
}
int main(int argc, char *argv[]) {
pthread_t threads[NUM_THREADS];
struct timeval start_time, end_time;
long t;
double single_thread_time, two_threads_time, four_threads_time;
// single thread
gettimeofday(&start_time, NULL);
calc_pi((void *));
gettimeofday(&end_time, NULL);
single_thread_time = (end_time.tv_sec - start_time.tv_sec) + (end_time.tv_usec - start_time.tv_usec) / 100000.;
printf("单线程时间:%.4f秒\n", single_thread_time);
// two threads
gettimeofday(&start_time, NULL);
for (t = ; t < 2; t++) {
pthread_create(&threads[t], NULL, calc_pi, (void *)t);
}
for (t = ; t < 2; t++) {
pthread_join(threads[t], NULL);
}
gettimeofday(&end_time, NULL);
two_threads_time = (end_time.tv_sec - start_time.tv_sec) + (end_time.tv_usec - start_time.tv_usec) / 100000.;
printf("2线程时间:%.4f秒\n", two_threads_time);
// four threads
gettimeofday(&start_time, NULL);
for (t = ; t < 4; t++) {
pthread_create(&threads[t], NULL, calc_pi, (void *)t);
}
for (t = ; t < 4; t++) {
pthread_join(threads[t], NULL);
}
gettimeofday(&end_time, NULL);
four_threads_time = (end_time.tv_sec - start_time.tv_sec) + (end_time.tv_usec - start_time.tv_usec) / 100000.;
printf("4线程时间:%.4f秒\n", four_threads_time);
return ;
}
```
在上面的代码中,我们定义了一个 calc_pi 函数来计算π的值,该函数接受一个线程 ID 参数,根据该参数计算出该线程需要计算的子任务范围,并将计算结果累加到全局变量 pi 中。在主函数中,我们先使用单线程计算π的值,并记录计算时间;然后使用两个线程和四个线程分别计算π的值,并记录计算时间。最后,我们按照要求输出不同线程计算π的时间。
希望这个回答能够帮助你!
相关推荐
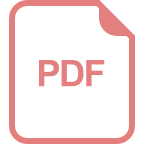
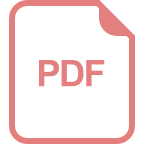














