.编写一个程序,该程序绘制一个5×9的网络,使用drawLine方法。//NetWork类
时间: 2023-09-10 17:05:37 浏览: 127
### 回答1:
下面是一个简单的Java程序,可以绘制一个5×9的网络:
```
import java.awt.*;
import javax.swing.*;
public class Network extends JPanel {
public void paintComponent(Graphics g) {
super.paintComponent(g);
Graphics2D g2 = (Graphics2D) g;
int width = getWidth();
int height = getHeight();
int x1, y1, x2, y2;
// draw vertical lines
for (int i = 0; i <= 5; i++) {
x1 = i * width / 5;
y1 = 0;
x2 = x1;
y2 = height;
g2.drawLine(x1, y1, x2, y2);
}
// draw horizontal lines
for (int i = 0; i <= 9; i++) {
x1 = 0;
y1 = i * height / 9;
x2 = width;
y2 = y1;
g2.drawLine(x1, y1, x2, y2);
}
}
public static void main(String[] args) {
JFrame frame = new JFrame("Network");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
Network network = new Network();
frame.add(network);
frame.setSize(400, 300);
frame.setVisible(true);
}
}
```
在这个程序中,我们创建了一个继承自JPanel的类Network,重写了其paintComponent方法来绘制网络。我们使用Graphics2D对象g2来绘制线条,首先绘制垂直线条,然后绘制水平线条。在main方法中,我们创建了一个JFrame窗口,并将Network对象添加到其中。最后,我们设置了窗口的大小并将其显示出来。
### 回答2:
NetWork类用于绘制一个5×9的网络,可以通过调用drawLine方法来实现。
下面是一个实现该功能的例子:
```java
import java.awt.*;
import javax.swing.*;
public class NetWork extends JPanel {
public void paintComponent(Graphics g) {
super.paintComponent(g);
int width = getWidth(); // 获取面板的宽度
int height = getHeight(); // 获取面板的高度
int cellWidth = width / 9; // 单元格的宽度
int cellHeight = height / 5; // 单元格的高度
// 绘制横向线条
for (int i = 0; i < 6; i++) {
int y = i * cellHeight;
g.drawLine(0, y, width, y); // 绘制线条
}
// 绘制纵向线条
for (int i = 0; i < 10; i++) {
int x = i * cellWidth;
g.drawLine(x, 0, x, height); // 绘制线条
}
}
public static void main(String[] args) {
JFrame frame = new JFrame("Network");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
NetWork network = new NetWork();
frame.add(network);
frame.setSize(500, 300);
frame.setVisible(true);
}
}
```
这段代码定义了一个NetWork类,继承自JPanel类。在paintComponent方法中,我们首先获取面板的宽度和高度,然后根据宽高计算出单元格的宽度和高度。接着使用两个for循环分别绘制横向和纵向的线条,使其形成一个5×9的网格。
在main方法中,我们创建了一个JFrame对象,并将NetWork对象添加到该窗口中,并设置窗口的大小和可见性。
### 回答3:
下面是一个用Java编写的程序,绘制一个5×9的网络:
```java
import java.awt.*;
import javax.swing.*;
public class NetWork extends JPanel {
@Override
public void paintComponent(Graphics g) {
super.paintComponent(g);
Graphics2D g2d = (Graphics2D) g;
int rows = 5;
int columns = 9;
int gap = 50;
for (int i = 0; i < rows + 1; i++) {
g2d.drawLine(0, i * gap, columns * gap, i * gap);
}
for (int i = 0; i < columns + 1; i++) {
g2d.drawLine(i * gap, 0, i * gap, rows * gap);
}
}
public static void main(String[] args) {
JFrame frame = new JFrame("Network");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setSize(500, 300);
frame.add(new NetWork());
frame.setVisible(true);
}
}
```
这个程序创建了一个名为`NetWork`的类,继承自`JPanel`。在`paintComponent`方法中,使用`Graphics2D`对象绘制了横向和纵向的线条来形成网络。程序以`main`方法为入口,创建了一个窗口,并将`NetWork`的实例添加到窗口中展示出来。
相关推荐
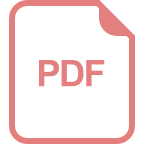
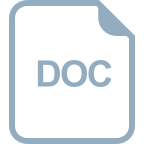














