java创建一个实现 turtle 图形 API 的类。编写一个递归方法来绘制希尔伯特曲线。代码实例
时间: 2023-06-10 20:04:22 浏览: 73
好的,让我来回答您的问题。
以下是实现 turtle 图形 API 的 Java 类,并编写了一个递归方法来绘制希尔伯特曲线的代码示例:
```java
import java.awt.Color;
import java.awt.Graphics;
import javax.swing.JFrame;
import javax.swing.JPanel;
public class Turtle {
private double x, y; // turtle position
private double angle; // turtle orientation
public Turtle(double x, double y, double angle) {
this.x = x;
this.y = y;
this.angle = angle;
}
public void forward(double dist) {
double newx = x + dist * Math.cos(Math.toRadians(angle));
double newy = y + dist * Math.sin(Math.toRadians(angle));
drawLine(x, y, newx, newy);
x = newx;
y = newy;
}
public void turnLeft(double delta) {
angle += delta;
}
public void turnRight(double delta) {
angle -= delta;
}
// Method to draw a line using Java's graphics library
private void drawLine(double x1, double y1, double x2, double y2) {
JFrame frame = new JFrame("Turtle Graphics");
JPanel panel = new JPanel() {
@Override
public void paintComponent(Graphics g) {
super.paintComponent(g);
g.setColor(Color.BLACK);
g.drawLine((int)x1, (int)y1, (int)x2, (int)y2);
}
};
frame.add(panel);
frame.setSize(500, 500);
frame.setVisible(true);
}
// Method to recursively draw a Hilbert curve
public void hilbertCurve(int level, double step, int direction) {
if (level == 0) {
return;
}
turnLeft(direction * 90);
hilbertCurve(level-1, step, -direction);
forward(step);
turnRight(direction * 90);
hilbertCurve(level-1, step, direction);
forward(step);
hilbertCurve(level-1, step, direction);
turnRight(direction * 90);
forward(step);
hilbertCurve(level-1, step, -direction);
turnLeft(direction * 90);
}
// Main method to create a Turtle object and draw a Hilbert curve
public static void main(String[] args) {
Turtle turtle = new Turtle(250, 250, 0);
turtle.hilbertCurve(6, 5, 1);
}
}
```
在这个示例中,我们创建了一个名为 Turtle 的类来实现 turtle 图形 API,并演示如何使用递归方法绘制希尔伯特曲线。在 hilbertCurve() 方法中,我们使用了四个递归调用来绘制一个更高级别的希尔伯特曲线,直到达到最低级别为止。为了绘制希尔伯特曲线,我们使用了 turnLeft()、turnRight() 和 forward() 方法来旋转和移动 turtle,从而绘制出曲线的各个部分。
在 main() 方法中,我们创建了一个 Turtle 对象,并调用 hilbertCurve() 方法来绘制希尔伯特曲线。通过调整 hilbertCurve() 方法中的参数,可以生成不同级别的希尔伯特曲线。
希望这个代码示例能够帮助您了解如何使用 Java 实现 turtle 图形 API 和递归方法绘制希尔伯特曲线。如果您还有其他问题,请随时提出,我会尽力回答。
相关推荐
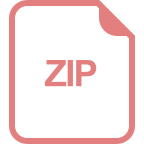
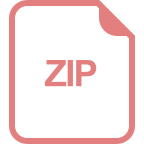














