java使用StdDraw创建一个Turtle实现 Turtle Graphics API 的功能
时间: 2023-06-08 16:08:50 浏览: 51
好的,很高兴为您解答这个问题。在Java中,您可以使用StdDraw库来创建一个类似于小海龟图形API的功能。步骤如下:
1. 创建一个Turtle类,其中包含了海龟的位置和朝向。
2. 使用StdDraw库中的绘图函数,在窗口中绘制出海龟的位置和朝向。
3. 实现Turtle类的运动功能,包括前进、旋转等操作,可以通过修改位置和朝向来实现。
下面是一个简单的示例代码,可以用于实现Turtle Graphics API的基本功能:
```
// Turtle类
class Turtle {
private double x, y; // 海龟的位置
private double angle; // 海龟的朝向
public Turtle(double x0, double y0, double a0) {
x = x0;
y = y0;
angle = a0;
}
// 前进函数
public void forward(double step) {
double x1 = x + step * Math.cos(Math.toRadians(angle));
double y1 = y + step * Math.sin(Math.toRadians(angle));
StdDraw.line(x, y, x1, y1); // 画线
x = x1;
y = y1;
}
// 角度旋转函数
public void turn(double delta) {
angle += delta;
}
}
// 测试代码
public class TurtleGraphics {
public static void main(String[] args) {
Turtle turtle = new Turtle(0.5, 0.5, 0.0); // 创建海龟对象
while (true) {
if (StdDraw.mousePressed()) {
// 在鼠标点击处向前移动一步
double x = StdDraw.mouseX();
double y = StdDraw.mouseY();
turtle.forward(0.01);
}
if (StdDraw.isKeyPressed('a')) {
// 左旋转
turtle.turn(15.0);
}
if (StdDraw.isKeyPressed('d')) {
// 右旋转
turtle.turn(-15.0);
}
}
}
}
```
注意,以上仅为示例代码,实现了一个基本的海龟图形API功能,您可以根据需求自行扩展和修改。希望能对您有所帮助。
相关推荐
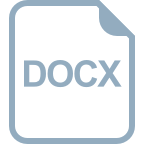














