java创建一个实现 turtle 图形 API 的类。编写一个递归方法来绘制希尔伯特曲线 使用StdDraw 类来画图。代码实例
时间: 2023-06-09 11:06:59 浏览: 91
很高兴和您进行对话!关于您的问题,我会尽力给您提供答案,以下是Java实现turtle图形API的类和绘制希尔伯特曲线的代码实例:
```java
import java.awt.Color;
public class Turtle {
private static final double RADS = Math.PI / 180.0; // 弧度制
private double x, y; // 初始位置为(0,0)
private double angle; // 初始角度为0度
private boolean penDown; // 笔是否落在画布上
public Turtle() {
this.x = 0;
this.y = 0;
this.angle = 0;
this.penDown = true; // 初始笔落在画布上
}
public void move(double distance) {
double radians = angle * RADS;
double newX = x + distance * Math.cos(radians);
double newY = y + distance * Math.sin(radians);
if (penDown) { // 如果笔落在画布上则绘制图形
drawLine(x, y, newX, newY);
}
x = newX;
y = newY;
}
public void turn(double delta) {
angle += delta;
}
public void penUp() {
penDown = false;
}
public void penDown() {
penDown = true;
}
private void drawLine(double x1, double y1, double x2, double y2) {
// 使用StdDraw类画图
StdDraw.line(x1, y1, x2, y2);
}
}
```
绘制希尔伯特曲线的递归方法如下:
```java
public class HilbertCurve {
public static void draw(Turtle turtle, int level, double step, int sign) {
if (level == 0) {
return;
}
turtle.turn(sign * 90);
draw(turtle, level - 1, step, -sign);
turtle.move(step);
turtle.turn(-sign * 90);
draw(turtle, level - 1, step, sign);
turtle.move(step);
draw(turtle, level - 1, step, sign);
turtle.turn(-sign * 90);
turtle.move(step);
draw(turtle, level - 1, step, -sign);
turtle.turn(sign * 90);
}
public static void main(String[] args) {
int level = 4; // 希尔伯特曲线的级别
double step = 0.5; // 每次移动的步长
int sign = 1; // 笔的方向
// 设置画布属性
StdDraw.setCanvasSize(800, 800);
StdDraw.setXscale(0, 1);
StdDraw.setYscale(0, 1);
StdDraw.enableDoubleBuffering();
Turtle turtle = new Turtle();
turtle.penDown();
StdDraw.setPenColor(Color.BLUE);
draw(turtle, level, step, sign);
StdDraw.show();
}
}
```
PS:使用StdDraw类需要导入algs4.jar包。
相关推荐
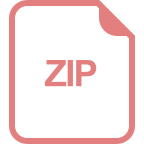














