model = models.resnet18(pretrained=False) model.fc = torch.nn.Linear(512, ???)
时间: 2023-06-24 19:07:35 浏览: 53
The number of output units in the `torch.nn.Linear` layer depends on the number of classes in your classification task. For example, if you have 10 classes, you would set the number of output units to 10:
```
model.fc = torch.nn.Linear(512, 10)
```
If you have a binary classification task, you would set the number of output units to 1:
```
model.fc = torch.nn.Linear(512, 1)
```
If you're doing regression instead of classification, you would set the number of output units to 1 (assuming you're predicting a single continuous value):
```
model.fc = torch.nn.Linear(512, 1)
```
相关问题
def resnet_34(): # Define the resnet model resnet = torchvision.models.resnet34(pretrained=True) # Update the fully connected layer of resnet with our current target of 10 desired outputs resnet.fc = torch.nn.Linear(resnet.fc.in_features, 10) # Initialize with xavier uniform torch.nn.init.xavier_uniform_(resnet.fc.weight) return resnet
这是一个使用PyTorch实现的ResNet-34模型,主要包括以下三个步骤:
1. 调用PyTorch内置的ResNet-34模型
```
resnet = torchvision.models.resnet34(pretrained=True)
```
2. 更新ResNet-34模型的全连接层,使其输出维度为10(这里假设是10分类问题)
```
resnet.fc = torch.nn.Linear(resnet.fc.in_features, 10)
```
3. 对全连接层的权重进行Xavier初始化
```
torch.nn.init.xavier_uniform_(resnet.fc.weight)
```
最终返回修改后的ResNet-34模型。
需要注意的是,这里使用了预训练的ResNet-34模型(pretrained=True),这意味着模型的所有权重已经在ImageNet数据集上进行了训练。因此,我们可以从预训练模型中学习到更好的特征表示,从而加速模型的训练和提高模型的准确率。
更改import torch import torchvision.models as models import torch.nn as nn import torch.nn.functional as F class eca_Resnet50(nn.Module): def init(self): super().init() self.model = models.resnet50(pretrained=True) self.model.avgpool = nn.AdaptiveAvgPool2d((1,1)) self.model.fc = nn.Linear(2048, 1000) self.eca = ECA_Module(2048, 8) def forward(self, x): x = self.model.conv1(x) x = self.model.bn1(x) x = self.model.relu(x) x = self.model.maxpool(x) x = self.model.layer1(x) x = self.model.layer2(x) x = self.model.layer3(x) x = self.model.layer4(x) x = self.eca(x) x = self.model.avgpool(x) x = torch.flatten(x, 1) x = self.model.fc(x) return x class ECA_Module(nn.Module): def init(self, channel, k_size=3): super(ECA_Module, self).init() self.avg_pool = nn.AdaptiveAvgPool2d(1) self.conv = nn.Conv1d(1, 1, kernel_size=k_size, padding=(k_size - 1) // 2, bias=False) self.sigmoid = nn.Sigmoid() def forward(self, x): b, c, _, _ = x.size() y = self.avg_pool(x) y = self.conv(y.squeeze(-1).transpose(-1,-2)).transpose(-1,-2).unsqueeze(-1) y = self.sigmoid(y) return x * y.expand_as(x) class ImageDenoising(nn.Module): def init(self): super().init() self.model = eca_Resnet50() self.conv1 = nn.Conv2d(3, 64, kernel_size=3, stride=1, padding=1) self.conv2 = nn.Conv2d(64, 64, kernel_size=3, stride=1, padding=1) self.conv3 = nn.Conv2d(64, 3, kernel_size=3, stride=1, padding=1) def forward(self, x): x = self.conv1(x) x = F.relu(x) x = self.conv2(x) x = F.relu(x) x = self.conv3(x) x = F.relu(x) return x,使最后输出为[16,1,50,50,]。
更改后的代码如下:
```
import torch
import torchvision.models as models
import torch.nn as nn
import torch.nn.functional as F
class eca_Resnet50(nn.Module):
def __init__(self):
super().__init__()
self.model = models.resnet50(pretrained=True)
self.model.avgpool = nn.AdaptiveAvgPool2d((1,1))
self.model.fc = nn.Linear(2048, 1000)
self.eca = ECA_Module(2048, 8)
def forward(self, x):
x = self.model.conv1(x)
x = self.model.bn1(x)
x = self.model.relu(x)
x = self.model.maxpool(x)
x = self.model.layer1(x)
x = self.model.layer2(x)
x = self.model.layer3(x)
x = self.model.layer4(x)
x = self.eca(x)
x = self.model.avgpool(x)
x = torch.flatten(x, 1)
x = self.model.fc(x)
return x
class ECA_Module(nn.Module):
def __init__(self, channel, k_size=3):
super().__init__()
self.avg_pool = nn.AdaptiveAvgPool2d(1)
self.conv = nn.Conv1d(1, 1, kernel_size=k_size, padding=(k_size - 1) // 2, bias=False)
self.sigmoid = nn.Sigmoid()
def forward(self, x):
b, c, _, _ = x.size()
y = self.avg_pool(x)
y = self.conv(y.squeeze(-1).transpose(-1,-2)).transpose(-1,-2).unsqueeze(-1)
y = self.sigmoid(y)
return x * y.expand_as(x)
class ImageDenoising(nn.Module):
def __init__(self):
super().__init__()
self.model = eca_Resnet50()
self.conv1 = nn.Conv2d(3, 64, kernel_size=3, stride=1, padding=1)
self.conv2 = nn.Conv2d(64, 64, kernel_size=3, stride=1, padding=1)
self.conv3 = nn.Conv2d(64, 1, kernel_size=3, stride=1, padding=1)
def forward(self, x):
x = self.conv1(x)
x = F.relu(x)
x = self.conv2(x)
x = F.relu(x)
x = self.conv3(x)
x = F.relu(x)
x = x.view(-1, 1, 50, 50)
return x
```
在修改的过程中,主要是将最后一层卷积层的输出通道数改为 1,并在 forward 方法中添加了 x.view(-1, 1, 50, 50),将输出的大小修改为 [16,1,50,50]。
相关推荐
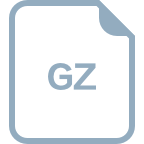
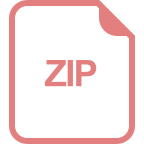












