Verilog case
时间: 2023-06-28 18:15:14 浏览: 53
Verilog中的case语句是一种控制结构,用于根据输入值执行不同的操作。它类似于C语言中的switch语句。
下面是一个简单的Verilog case语句的示例:
```verilog
module example(input [1:0] sel, output reg [3:0] out);
always @(sel) begin
case(sel)
2'b00: out = 4'b0000;
2'b01: out = 4'b0001;
2'b10: out = 4'b0010;
2'b11: out = 4'b0011;
default: out = 4'bXXXX;
endcase
end
endmodule
```
在这个例子中,输入sel是一个2位二进制数字,输出out是一个4位二进制数字。根据输入sel的值,case语句将out赋值为不同的值。如果输入sel的值与任何一个case标签都不匹配,则执行default标签。
请注意,case语句是一个阻塞式的结构,它会在每次输入sel发生变化时执行。在这个例子中,always块的敏感列表包括sel,因此当输入sel发生变化时,case语句将重新执行,并根据新的输入sel值更新输出out。
相关问题
verilog case
Verilog中的case语句用于根据选择变量的不同值执行不同的操作。它类似于C语言中的switch语句。
下面是一个Verilog中case语句的示例:
```verilog
module my_module (
input [1:0] sel,
output reg [3:0] out
);
always @(*) begin
case(sel)
2'b00: out = 4'b0001;
2'b01: out = 4'b0010;
2'b10: out = 4'b0100;
2'b11: out = 4'b1000;
default: out = 4'b1111;
endcase
end
endmodule
```
在上面的示例中,我们定义了一个模块,该模块具有两个输入(sel)和一个输出(out)。当sel的值为00、01、10或11时,out的值将分别设置为0001、0010、0100或1000。如果sel的值不属于这些情况,则out的值将被设置为1111。
需要注意的是,在Verilog中,case语句中的选择变量必须是有限的值集合,否则编译器会产生警告。
verilog case语句
Verilog中的case语句是一种多分支选择语句,用于根据不同的条件执行不同的操作。它的基本语法如下:
```verilog
case (expression)
value1: statement1;
value2: statement2;
...
default: statementN;
endcase
```
其中,expression是一个表达式,用于确定要执行的分支。每个value是一个常量或表达式,用于与expression进行比较。如果expression的值与某个value匹配,则执行相应的statement。如果expression的值与任何value都不匹配,则执行default后面的statement。
以下是一个示例,演示了Verilog中case语句的用法:
```verilog
module example;
reg [1:0] sel;
reg [3:0] out;
always @*
case (sel)
2'b00: out = 4'b0000;
2'b01: out = 4'b0001;
2'b10: out = 4'b0010;
2'b11: out = 4'b0011;
default: out = 4'b1111;
endcase
endmodule
```
在上面的例子中,根据sel的值,case语句将out赋予不同的值。如果sel为00,则out为0000;如果sel为01,则out为0001;如果sel为10,则out为0010;如果sel为11,则out为0011;如果sel的值与任何value都不匹配,则out为1111。
相关推荐
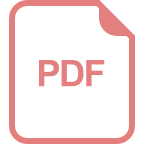
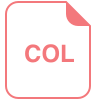
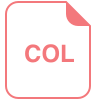
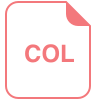
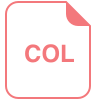








