import numpy as np from scipy.optimize import minimize # 定义目标函数 def objective(x): return 2*(x[0]**2)+2*(x[1]**2)-2*x[0]*x[1]-4*x[0]-6*x[1] # 定义目标函数的梯度 def gradient(x): return np.array([4*x[0]-2*x[1]-4, 4*x[0]-2*x[1]-6]) # 定义约束条件 def constraint1(x): return -x[0] - x[1] +2 def constraint2(x): return -x[0] - x[1] - 5 # 定义初始点 x0 = np.array([0, 0]) # 定义约束条件字典 constraints = [{'type': 'ineq', 'fun': constraint1}, {'type': 'ineq', 'fun': constraint2}] # 定义迭代过程记录列表 iteration_history = [] # 定义迭代回调函数 def callback(xk): iteration_history.append(xk) # 使用梯度投影法求解优化问题 result = minimize(objective, x0, method='COBYLA', jac=gradient, constraints=constraints, callback=callback, options={'maxiter': 100}) # 输出结果 print('拟合结果:') print('最优解:', result.x) print('目标函数值:', result.fun) print('约束条件1:', constraint1(result.x)) print('约束条件2:', constraint2(result.x)) # 绘制迭代过程图 import matplotlib.pyplot as plt iteration_history = np.array(iteration_history) plt.plot(iteration_history[:, 0], iteration_history[:, 1], marker='o') plt.xlabel('x1') plt.ylabel('x2') plt.title('Iteration History') plt.show()以上代码出现IndexError: too many indices for array: array is 1-dimensional, but 2 were indexed报错该如何解决
时间: 2024-03-17 15:47:11 浏览: 41
这个错误可能是由于在迭代回调函数中使用 np.array() 将单个数字转换为数组所致。在这种情况下,可以使用 np.append() 函数将单个数字添加到数组中。以下是修改回调函数的示例代码:
```
def callback(xk):
iteration_history.append(xk.tolist())
```
这将将每个迭代步骤的坐标作为列表添加到 iteration_history 数组中。然后,您可以使用以下代码来绘制迭代图:
```
iteration_history = np.array(iteration_history)
plt.plot(iteration_history[:, 0], iteration_history[:, 1], marker='o')
plt.xlabel('x1')
plt.ylabel('x2')
plt.title('Iteration History')
plt.show()
```
这应该解决这个问题。
相关问题
import numpy as np from scipy.stats import norm from scipy.optimize import minimize # 添加优化模块 #样本点 X1 = -1. X2 = 2. X1 = -1. X2 = 2. #构造似然函数 def likelihood(r): coef = 1. / (2. * np.pi * np.sqrt(1. - r2)) exp_term = -0.5 * (X1_2 + X2_2 - 2*r*X1*X2) / (1.0 - r**2) return coef * np.exp(exp_term) #最大化似然函数,得到r的最大似然估计值 result = minimize(lambda x: -likelihood(x), 0.) # 优化目标函数 r_mle = result.x print("r的最大似然估计值:", r_mle)优化这段代码,并解释每行意思
```
import numpy as np
from scipy.stats import norm
from scipy.optimize import minimize
# 定义两个样本点
X1 = -1.
X2 = 2.
# 构造似然函数
def likelihood(r):
r2 = r**2
X1_2 = X1**2
X2_2 = X2**2
coef = 1. / (2. * np.pi * np.sqrt(1. - r2)) # 计算系数
exp_term = -0.5 * (X1_2 + X2_2 - 2*r*X1*X2) / (1.0 - r**2) # 计算指数项
return coef * np.exp(exp_term) # 返回似然函数值
# 最大化似然函数,得到r的最大似然估计值
result = minimize(lambda x: -likelihood(x), 0.) # 优化目标函数,使用minimize函数求解最小值,这里采用了lambda表达式来定义目标函数
r_mle = result.x # 取得最小值,即最大似然估计值
# 输出结果
print("r的最大似然估计值:", r_mle)
```
这段代码的作用是:给定两个样本点X1和X2,通过最大化似然函数来估计它们之间的相关系数r的值。具体步骤如下:
1. 导入所需的Python库:`numpy`、`scipy.stats`和`scipy.optimize`
2. 定义两个样本点X1和X2
3. 定义似然函数`likelihood(r)`,其中`r`为相关系数,该函数返回给定相关系数下的似然函数值
4. 使用`scipy.optimize.minimize`函数优化似然函数,求解最大似然估计值。这里采用了lambda表达式来定义目标函数(即似然函数的相反数),0.为初始猜测值。
5. 取得最小值,即最大似然估计值。
6. 输出结果。
以下代码总是提示错误ZeroDivisionError: float division by zero,怎么解决import numpy as np from scipy.optimize import minimize_scalar # 定义似然函数 def likelihood(r): return -np.log(2 * np.pi) - 0.5 * np.log(1 - r**2) - 0.5 * (1**2 - 2 * r * 1 * 2 + 2**2)/ (1 - r**2) bounds = (0.5, 0.7) # 最大似然估计 result = minimize_scalar(lambda r: -likelihood(r),method='brent',bounds=bounds) print(result)这段代码总是提示错误
这个错误的原因在于在计算似然函数时,分母可能为零,导致了除以零错误。
你可以尝试以下两种方法来解决这个问题:
方法一:
在计算似然函数时,将分母加上一个非常小的数,例如 `1e-10`,以避免分母为零的情况。修改后的代码如下:
```python
import numpy as np
from scipy.optimize import minimize_scalar
# 定义似然函数
def likelihood(r):
return -np.log(2 * np.pi) - 0.5 * np.log(1 - r**2 + 1e-10) - 0.5 * (1**2 - 2 * r * 1 * 2 + 2**2)/ (1 - r**2 + 1e-10)
bounds = (0.5, 0.7)
# 最大似然估计
result = minimize_scalar(lambda r: -likelihood(r), method='brent', bounds=bounds)
print(result)
```
方法二:
检查一下分母是否为零的情况,并在这种情况下返回一个非常大的数,例如 `1e10`,以避免除以零错误。修改后的代码如下:
```python
import numpy as np
from scipy.optimize import minimize_scalar
# 定义似然函数
def likelihood(r):
if 1 - r**2 == 0:
return 1e10
return -np.log(2 * np.pi) - 0.5 * np.log(1 - r**2) - 0.5 * (1**2 - 2 * r * 1 * 2 + 2**2)/ (1 - r**2)
bounds = (0.5, 0.7)
# 最大似然估计
result = minimize_scalar(lambda r: -likelihood(r), method='brent', bounds=bounds)
print(result)
```
这两种方法都可以避免除以零错误,但是方法一更加简单,并且适用于更广泛的情况。
相关推荐
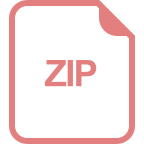
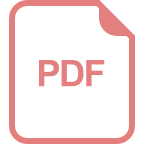
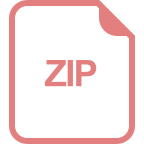













