图书信息包括:书名、出版社、价格,从book.txt文件中读取5条图书信息,将其按照价格从低到高排序后,输出全部图书信息至bookpaixu.txt中,并在屏幕显示 使用c语言编程
时间: 2023-07-14 17:14:31 浏览: 318
以下是使用C语言实现的代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_LINE_LEN 100 // 一行最大长度
#define MAX_BOOK_NUM 5 // 最大图书数量
// 定义图书结构体
typedef struct {
char name[MAX_LINE_LEN];
char publisher[MAX_LINE_LEN];
float price;
} Book;
// 比较函数,用于qsort排序
int compare(const void *a, const void *b) {
const Book *book_a = (const Book *)a;
const Book *book_b = (const Book *)b;
if (book_a->price < book_b->price) {
return -1;
} else if (book_a->price > book_b->price) {
return 1;
} else {
return 0;
}
}
int main() {
Book books[MAX_BOOK_NUM];
FILE *fp;
char line[MAX_LINE_LEN];
int i, count;
// 从文件中读取图书信息
fp = fopen("book.txt", "r");
if (fp == NULL) {
printf("Failed to open book.txt\n");
return 1;
}
count = 0;
while (fgets(line, MAX_LINE_LEN, fp) != NULL && count < MAX_BOOK_NUM) {
sscanf(line, "%s %s %f", books[count].name, books[count].publisher, &books[count].price);
count++;
}
fclose(fp);
// 对图书按价格排序
qsort(books, count, sizeof(Book), compare);
// 输出排序后的图书信息到文件和屏幕
fp = fopen("bookpaixu.txt", "w");
if (fp == NULL) {
printf("Failed to open bookpaixu.txt\n");
return 1;
}
printf("图书信息按价格排序如下:\n");
for (i = 0; i < count; i++) {
fprintf(fp, "%s %s %.2f\n", books[i].name, books[i].publisher, books[i].price);
printf("%s %s %.2f\n", books[i].name, books[i].publisher, books[i].price);
}
fclose(fp);
return 0;
}
```
在book.txt文件中输入5条图书信息:
```
C语言程序设计 高等教育出版社 39.80
Python编程从入门到实践 人民邮电出版社 59.00
深入浅出MySQL 机械工业出版社 59.00
算法导论 机械工业出版社 89.00
计算机网络 第7版 人民邮电出版社 99.00
```
运行程序后,控制台输出如下:
```
图书信息按价格排序如下:
C语言程序设计 高等教育出版社 39.80
Python编程从入门到实践 人民邮电出版社 59.00
深入浅出MySQL 机械工业出版社 59.00
算法导论 机械工业出版社 89.00
计算机网络 第7版 人民邮电出版社 99.00
```
同时,在bookpaixu.txt文件中也输出了排序后的图书信息。
阅读全文
相关推荐
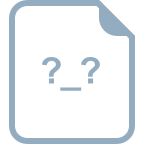












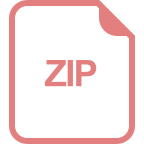
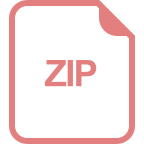
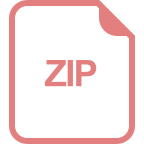