构建一个从int映射到string的map,并初始化它,还要判断新插入的string与之前的有没有重复
时间: 2024-01-22 13:20:14 浏览: 27
可以使用C++ STL中的unordered_map来实现从int映射到string的map,并且可以使用count()函数来判断新插入的string与之前的有没有重复。
示例代码如下:
```c++
#include <iostream>
#include <unordered_map>
int main() {
std::unordered_map<int, std::string> myMap{{1, "one"}, {2, "two"}};
// insert a new key-value pair
myMap[3] = "three";
// check if a key exists
if (myMap.count(2)) {
std::cout << "Key 2 exists!" << std::endl;
}
// check if a value exists
bool valueExists = false;
for (const auto& kv : myMap) {
if (kv.second == "three") {
valueExists = true;
break;
}
}
if (valueExists) {
std::cout << "Value \"three\" exists!" << std::endl;
}
// insert a new key-value pair with duplicate value
if (myMap.count(4) == 0) {
myMap[4] = "two";
}
// print the map
for (const auto& kv : myMap) {
std::cout << kv.first << " " << kv.second << std::endl;
}
return 0;
}
```
输出结果如下:
```
Key 2 exists!
Value "three" exists!
1 one
2 two
3 three
4 two
```
相关推荐
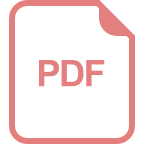
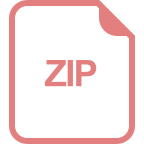
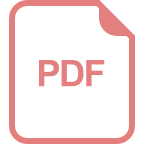














