C++设计模式,并给出例程
时间: 2023-07-13 22:05:09 浏览: 50
C++设计模式是一种被广泛使用的面向对象编程思想,它提供了一种优秀的设计方案,以解决复杂的软件开发问题。以下是常见的几种C++设计模式及其相应的例程。
1. 工厂模式(Factory Pattern)
工厂模式是一种创建型模式,它提供了一种将对象实例化的方式,而不需要直接使用 new 运算符来创建对象。以下是一个简单的工厂模式的例程:
```
#include <iostream>
using namespace std;
class Product {
public:
virtual void use() = 0;
};
class ConcreteProductA : public Product {
public:
void use() {
cout << "Use concrete product A." << endl;
}
};
class ConcreteProductB : public Product {
public:
void use() {
cout << "Use concrete product B." << endl;
}
};
class Factory {
public:
virtual Product* createProduct() = 0;
};
class ConcreteFactoryA : public Factory {
public:
Product* createProduct() {
return new ConcreteProductA();
}
};
class ConcreteFactoryB : public Factory {
public:
Product* createProduct() {
return new ConcreteProductB();
}
};
int main() {
Factory* factoryA = new ConcreteFactoryA();
Product* productA = factoryA->createProduct();
productA->use();
Factory* factoryB = new ConcreteFactoryB();
Product* productB = factoryB->createProduct();
productB->use();
delete productA;
delete productB;
delete factoryA;
delete factoryB;
return 0;
}
```
2. 单例模式(Singleton Pattern)
单例模式是一种创建型模式,它确保一个类只有一个实例,并提供了全局访问点。以下是一个简单的单例模式的例程:
```
#include <iostream>
using namespace std;
class Singleton {
private:
static Singleton* instance;
Singleton() {}
public:
static Singleton* getInstance() {
if (instance == NULL) {
instance = new Singleton();
}
return instance;
}
void sayHello() {
cout << "Hello, world!" << endl;
}
};
Singleton* Singleton::instance = NULL;
int main() {
Singleton* singleton = Singleton::getInstance();
singleton->sayHello();
delete singleton;
return 0;
}
```
3. 装饰器模式(Decorator Pattern)
装饰器模式是一种结构型模式,它允许将行为动态地添加到对象中。以下是一个简单的装饰器模式的例程:
```
#include <iostream>
using namespace std;
class Component {
public:
virtual void operation() = 0;
};
class ConcreteComponent : public Component {
public:
void operation() {
cout << "Concrete component operation." << endl;
}
};
class Decorator : public Component {
protected:
Component* component;
public:
Decorator(Component* component) {
this->component = component;
}
void operation() {
component->operation();
}
};
class ConcreteDecoratorA : public Decorator {
public:
ConcreteDecoratorA(Component* component) : Decorator(component) {}
void operation() {
Decorator::operation();
cout << "Concrete decorator A operation." << endl;
}
};
class ConcreteDecoratorB : public Decorator {
public:
ConcreteDecoratorB(Component* component) : Decorator(component) {}
void operation() {
Decorator::operation();
cout << "Concrete decorator B operation." << endl;
}
};
int main() {
Component* component = new ConcreteComponent();
Decorator* decoratorA = new ConcreteDecoratorA(component);
Decorator* decoratorB = new ConcreteDecoratorB(decoratorA);
decoratorB->operation();
delete decoratorB;
delete decoratorA;
delete component;
return 0;
}
```
4. 观察者模式(Observer Pattern)
观察者模式是一种行为型模式,它定义了对象之间的一对多依赖关系,当一个对象状态发生改变时,所有依赖它的对象都会得到通知并自动更新。以下是一个简单的观察者模式的例程:
```
#include <iostream>
#include <vector>
using namespace std;
class Observer {
public:
virtual void update(int value) = 0;
};
class Subject {
private:
vector<Observer*> observers;
public:
void attach(Observer* observer) {
observers.push_back(observer);
}
void detach(Observer* observer) {
for (vector<Observer*>::iterator it = observers.begin(); it != observers.end(); it++) {
if (*it == observer) {
observers.erase(it);
break;
}
}
}
void notify(int value) {
for (vector<Observer*>::iterator it = observers.begin(); it != observers.end(); it++) {
(*it)->update(value);
}
}
};
class ConcreteObserverA : public Observer {
public:
void update(int value) {
cout << "Concrete observer A received update with value " << value << "." << endl;
}
};
class ConcreteObserverB : public Observer {
public:
void update(int value) {
cout << "Concrete observer B received update with value " << value << "." << endl;
}
};
int main() {
Subject* subject = new Subject();
Observer* observerA = new ConcreteObserverA();
Observer* observerB = new ConcreteObserverB();
subject->attach(observerA);
subject->attach(observerB);
subject->notify(42);
subject->detach(observerA);
subject->notify(1337);
delete observerB;
delete observerA;
delete subject;
return 0;
}
```
相关推荐
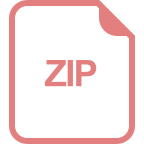
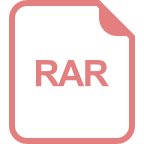














