用C语言输入数个字符建立字符顺序表,在顺序表上练习①求表长度;②按序号查找;③插入元素 的操作。
时间: 2023-05-23 19:01:22 浏览: 90
答案:
① 建立字符顺序表:
```c
#define MAXSIZE 100 //固定长度
typedef struct
{
char ch[MAXSIZE];
int length;
}SqString;
void createString(SqString& s, char cstr[])
{
int len = strlen(cstr);
for(int i=0; i<len; i++)
{
s.ch[i] = cstr[i];
}
s.length = len;
}
```
② 求表长度:
```c
int getLength(SqString s)
{
return s.length;
}
```
③ 按序号查找:
```c
char getElem(SqString s, int i)
{
if(i<1 || i>s.length) exit(EXIT_FAILURE);
return s.ch[i-1];
}
```
④ 插入元素:
```c
void insertElem(SqString& s, int i, char e)
{
if(i<1 || i>s.length+1) exit(EXIT_FAILURE);
if(s.length >= MAXSIZE) exit(EXIT_FAILURE);
for(int j=s.length; j>=i; j--)
{
s.ch[j] = s.ch[j-1];
}
s.ch[i-1] = e;
s.length++;
}
```
请注意,这里给出的只是一个基本的参考实现,实际上可能需要根据具体的应用场景进行调整或优化。如果需要更具体的实现细节或优化建议,可以查阅相关的参考资料或咨询专业人士。
相关推荐
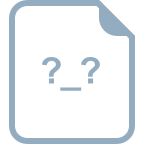














