编写一个贪吃蛇游戏
时间: 2023-07-01 17:10:24 浏览: 51
好的,我可以使用 Python 编写一个贪吃蛇游戏。
首先,我们需要导入必要的模块,包括 `pygame` 和 `random`:
```python
import pygame
import random
```
然后,我们需要定义一些常量,包括窗口大小、蛇的初始位置、蛇身体的颜色等:
```python
WIDTH = 500
HEIGHT = 500
FPS = 10
# Snake settings
snake_pos = [(WIDTH // 2, HEIGHT // 2)]
snake_size = 10
snake_color = (255, 255, 255)
```
接下来,我们需要初始化 Pygame,创建一个窗口,并设置一些基本的游戏参数:
```python
# Initialize Pygame
pygame.init()
pygame.mixer.init()
screen = pygame.display.set_mode((WIDTH, HEIGHT))
pygame.display.set_caption("Snake Game")
clock = pygame.time.Clock()
```
然后,我们需要定义两个函数:一个用于生成食物的随机位置,另一个用于检查蛇是否碰到了边界或自己:
```python
def generate_food():
x = random.randrange(0, WIDTH, snake_size)
y = random.randrange(0, HEIGHT, snake_size)
return (x, y)
def check_collision():
head = snake_pos[-1]
if head[0] < 0 or head[0] >= WIDTH or head[1] < 0 or head[1] >= HEIGHT:
return True
for pos in snake_pos[:-1]:
if head == pos:
return True
return False
```
接下来,我们需要定义主循环。在主循环中,我们需要检测事件,更新蛇的位置,绘制蛇和食物,检查碰撞等:
```python
# Game loop
running = True
food_pos = generate_food()
while running:
# Keep running at the right speed
clock.tick(FPS)
# Process input (events)
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
# Update
snake_pos = snake_pos[-1:] + snake_pos[:-1]
head = snake_pos[-1]
if direction == "UP":
head = (head[0], head[1] - snake_size)
elif direction == "DOWN":
head = (head[0], head[1] + snake_size)
elif direction == "LEFT":
head = (head[0] - snake_size, head[1])
elif direction == "RIGHT":
head = (head[0] + snake_size, head[1])
snake_pos[-1] = head
if head == food_pos:
snake_pos.insert(0, food_pos)
food_pos = generate_food()
if check_collision():
running = False
# Draw / render
screen.fill((0, 0, 0))
for pos in snake_pos:
pygame.draw.rect(screen, snake_color, (pos[0], pos[1], snake_size, snake_size))
pygame.draw.rect(screen, (255, 0, 0), (food_pos[0], food_pos[1], snake_size, snake_size))
# Flip the display
pygame.display.flip()
```
最后,我们需要清理 Pygame 并退出程序:
```python
# Quit Pygame
pygame.quit()
```
完整的代码如下:
相关推荐
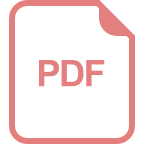
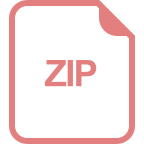
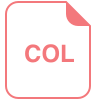
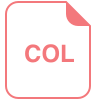
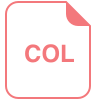
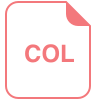
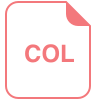








