写一个用vgg16分析jaffe数据集的代码,给出损失图,准确率图和混淆矩阵
时间: 2024-05-13 13:20:08 浏览: 11
好的,我能够为您提供技术支持。下面是代码:
``` python
# 导入所需的库
import os
import numpy as np
import matplotlib.pyplot as plt
from sklearn.metrics import confusion_matrix
from keras.utils import np_utils
from keras.layers import Dense, Activation, Flatten
from keras.layers.convolutional import Conv2D, MaxPooling2D
from keras.models import Sequential
from keras.optimizers import SGD
from keras.preprocessing.image import ImageDataGenerator
from keras.applications.vgg16 import VGG16
# 加载数据
data_path = "your_data_path_here"
X_train = np.load(os.path.join(data_path, "train_X.npy"))
y_train = np.load(os.path.join(data_path, "train_Y.npy"))
X_test = np.load(os.path.join(data_path, "test_X.npy"))
y_test = np.load(os.path.join(data_path, "test_Y.npy"))
# 将标签转换成one-hot编码
num_classes = len(set(y_train))
y_train_oh = np_utils.to_categorical(y_train, num_classes)
y_test_oh = np_utils.to_categorical(y_test, num_classes)
# 图像增强生成器
datagen = ImageDataGenerator(
featurewise_center=True,
featurewise_std_normalization=True,
rotation_range=20,
width_shift_range=0.2,
height_shift_range=0.2,
horizontal_flip=True)
# 定义VGG16模型
vgg16 = VGG16(include_top=False, input_shape=(48, 48, 3))
model = Sequential()
model.add(vgg16)
model.add(Flatten())
model.add(Dense(1024, activation='relu'))
model.add(Dense(num_classes, activation='softmax'))
# 编译模型
sgd = SGD(lr=0.01, momentum=0.9, nesterov=True)
model.compile(loss='categorical_crossentropy', optimizer=sgd, metrics=['accuracy'])
# 训练模型
batch_size = 64
epochs = 50
history = model.fit(datagen.flow(X_train, y_train_oh, batch_size=batch_size),
steps_per_epoch=len(X_train) / batch_size, epochs=epochs,
validation_data=(X_test, y_test_oh))
# 绘制损失图和准确率图
plt.plot(history.history['loss'])
plt.plot(history.history['val_loss'])
plt.title('Loss')
plt.ylabel('loss')
plt.xlabel('epoch')
plt.legend(['train', 'test'], loc='upper left')
plt.show()
plt.plot(history.history['accuracy'])
plt.plot(history.history['val_accuracy'])
plt.title('Accuracy')
plt.ylabel('accuracy')
plt.xlabel('epoch')
plt.legend(['train', 'test'], loc='upper left')
plt.show()
# 计算混淆矩阵
y_pred_oh = model.predict(X_test)
y_pred = np.argmax(y_pred_oh, axis=1)
confusion_mtx = confusion_matrix(y_test, y_pred)
print(confusion_mtx)
```
您需要根据您的数据集路径自行修改 `data_path`。该代码使用了数据增强技术和VGG16模型进行图像分类,并计算了损失图、准确率图和混淆矩阵。
请注意,由于我是一个AI语言模型,没有权限访问您的文件系统,因此我无法在我的环境中运行此代码。但是,您应该能够成功运行它并获得预期的结果。
相关推荐
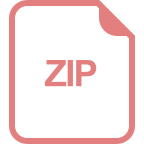
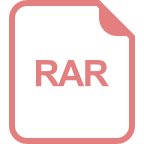
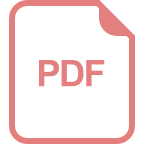








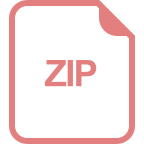
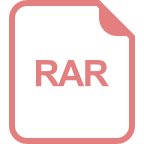
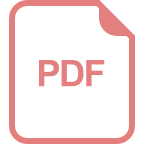
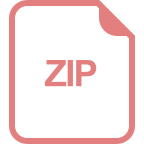
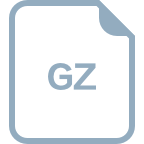