label smoothing在pytorch中具体怎么应用
时间: 2024-05-09 16:21:08 浏览: 72
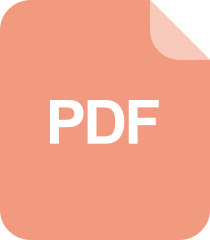
在PyTorch中使用标签平滑正则化的问题
在PyTorch中,可以通过定义一个自定义的损失函数来应用标签平滑(Label Smoothing)方法。以下是一个示例代码:
```python
import torch
import torch.nn as nn
class LabelSmoothingLoss(nn.Module):
def __init__(self, smoothing=0.0):
super(LabelSmoothingLoss, self).__init__()
self.smoothing = smoothing
self.confidence = 1.0 - smoothing
def forward(self, x, target):
# 将目标标签转为one-hot编码
target = target.unsqueeze(1)
one_hot = torch.zeros_like(x).scatter(1, target, 1)
# 平滑标签
smooth_target = one_hot * self.confidence + (1 - one_hot) * self.smoothing / (x.size(1) - 1)
# 计算交叉熵损失
log_probs = nn.functional.log_softmax(x, dim=1)
loss = torch.sum(-smooth_target * log_probs, dim=1)
return loss.mean()
```
在这个自定义的损失函数中,我们首先将目标标签转为one-hot编码,并使用PyTorch的`scatter`方法实现这个过程。然后,我们根据标签平滑的公式对one-hot编码进行平滑操作。最后,我们使用PyTorch的`log_softmax`方法计算log概率,并使用平滑后的标签计算交叉熵损失。
使用这个自定义的损失函数时,可以像使用其他PyTorch损失函数一样将其传递给模型的训练过程中,例如:
```python
model = MyModel()
criterion = LabelSmoothingLoss(smoothing=0.1)
optimizer = torch.optim.SGD(model.parameters(), lr=0.01)
for epoch in range(num_epochs):
for batch in data_loader:
inputs, targets = batch
outputs = model(inputs)
loss = criterion(outputs, targets)
optimizer.zero_grad()
loss.backward()
optimizer.step()
```
在这个训练过程中,我们将使用标签平滑损失函数来计算模型的损失,并使用随机梯度下降(SGD)算法来更新模型的参数。
阅读全文
相关推荐

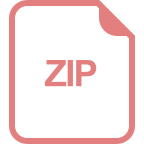
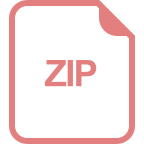
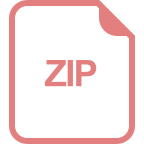
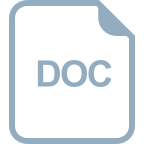
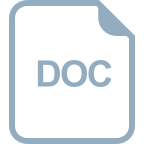
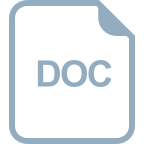










