如何在DQN网络结构中实现噪声网络,用代码实现
时间: 2024-03-26 11:38:33 浏览: 21
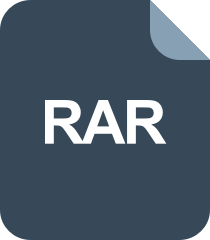
DQN-by-keras-master.rar
在DQN网络结构中实现噪声网络可以提高探索性,增加智能体的行动多样性,从而提高策略的鲁棒性和性能。以下是一个基于PyTorch实现的噪声网络的示例代码:
```python
import torch.nn as nn
import torch.nn.functional as F
import torch.optim as optim
import numpy as np
class NoisyLinear(nn.Module):
def __init__(self, in_features, out_features, sigma_init=0.5):
super(NoisyLinear, self).__init__()
self.in_features = in_features
self.out_features = out_features
self.sigma_init = sigma_init
self.weight = nn.Parameter(torch.Tensor(out_features, in_features))
self.bias = nn.Parameter(torch.Tensor(out_features))
self.register_buffer('epsilon_weight', torch.zeros(out_features, in_features))
self.register_buffer('epsilon_bias', torch.zeros(out_features))
self.reset_parameters()
self.reset_noise()
def reset_parameters(self):
stdv = 1.0 / np.sqrt(self.in_features)
self.weight.data.uniform_(-stdv, stdv)
self.bias.data.uniform_(-stdv, stdv)
def reset_noise(self):
epsilon_weight = torch.randn(self.out_features, self.in_features)
epsilon_bias = torch.randn(self.out_features)
self.epsilon_weight.copy_(epsilon_weight)
self.epsilon_bias.copy_(epsilon_bias)
def forward(self, input):
if not self.training:
return F.linear(input, self.weight, self.bias)
weight = self.weight + self.sigma_init * self.epsilon_weight
bias = self.bias + self.sigma_init * self.epsilon_bias
return F.linear(input, weight, bias)
class DQN(nn.Module):
def __init__(self, num_inputs, num_actions, sigma_init=0.5):
super(DQN, self).__init__()
self.num_inputs = num_inputs
self.num_actions = num_actions
self.sigma_init = sigma_init
self.fc1 = NoisyLinear(num_inputs, 128, sigma_init)
self.fc2 = NoisyLinear(128, 128, sigma_init)
self.fc3 = NoisyLinear(128, num_actions, sigma_init)
def forward(self, state):
x = F.relu(self.fc1(state))
x = F.relu(self.fc2(x))
x = self.fc3(x)
return x
```
在这个实现中,我们定义了一个`NoisyLinear`的类,它继承自PyTorch的`nn.Module`类,这个类的作用是在前向传播中添加噪声,从而实现噪声网络。在`NoisyLinear`类的`__init__`方法中,我们定义了网络的参数(权重和偏置)以及噪声参数(`epsilon_weight`和`epsilon_bias`)。`reset_parameters`方法用于初始化参数,`reset_noise`方法用于初始化噪声参数。`forward`方法用于前向传播,其中如果网络处于训练状态,就将权重和偏置加上噪声后再进行线性运算。
在`DQN`类中,我们使用了三个`NoisyLinear`层来构建网络。在前向传播中,我们首先通过一个`fc1`层将输入状态转换为128维的特征向量,然后通过一个`fc2`层进一步提取特征,最后通过一个`fc3`层将特征向量映射为动作空间的Q值。
阅读全文
相关推荐
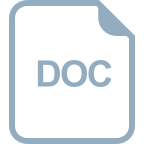
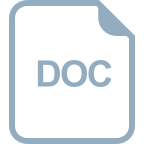
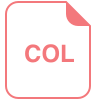
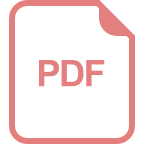
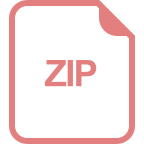
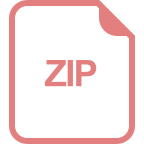
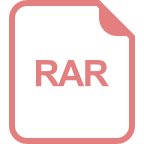
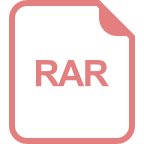
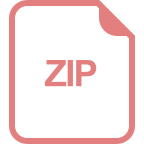
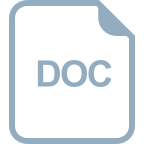
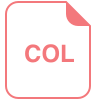
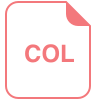
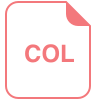
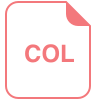
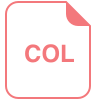
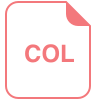
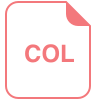
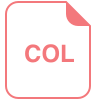