不同空间直角坐标系间坐标转换不用库自己写c#
时间: 2023-07-27 15:19:51 浏览: 118
进行不同空间直角坐标系间坐标转换的一种方法是使用矩阵乘法,通过将坐标与转换矩阵相乘来实现坐标的转换。
以下是一个示例代码:
```csharp
// 定义要进行转换的坐标
double x = 1;
double y = 2;
double z = 3;
// 定义空间1和空间2的坐标系
double[,] space1 = new double[,] { { 1, 0, 0 }, { 0, 1, 0 }, { 0, 0, 1 } };
double[,] space2 = new double[,] { { 0.5, 0, 0 }, { 0, 0.5, 0 }, { 0, 0, 0.5 } };
// 定义一个3x1的矩阵表示要进行转换的坐标
double[,] pointMatrix = new double[,] { { x }, { y }, { z } };
// 将坐标从空间1转换到空间2
double[,] transformedPointMatrix = Multiply(space2, Multiply(space1, pointMatrix));
// 输出转换后的坐标
double transformedX = transformedPointMatrix[0, 0];
double transformedY = transformedPointMatrix[1, 0];
double transformedZ = transformedPointMatrix[2, 0];
Console.WriteLine($"({transformedX}, {transformedY}, {transformedZ})");
// 定义矩阵乘法函数
static double[,] Multiply(double[,] matrix1, double[,] matrix2)
{
int m1 = matrix1.GetLength(0);
int n1 = matrix1.GetLength(1);
int m2 = matrix2.GetLength(0);
int n2 = matrix2.GetLength(1);
if (n1 != m2)
{
throw new ArgumentException("The number of columns in the first matrix must equal the number of rows in the second matrix.");
}
double[,] result = new double[m1, n2];
for (int i = 0; i < m1; i++)
{
for (int j = 0; j < n2; j++)
{
double sum = 0;
for (int k = 0; k < n1; k++)
{
sum += matrix1[i, k] * matrix2[k, j];
}
result[i, j] = sum;
}
}
return result;
}
```
在这个示例中,我们定义了要进行转换的坐标(x、y、z),以及空间1和空间2的坐标系(分别为space1和space2)。然后,在定义一个3x1的矩阵表示要进行转换的坐标,使用矩阵乘法将其从空间1转换到空间2,最后输出转换后的坐标。
我们还定义了一个矩阵乘法函数Multiply,它接受两个矩阵并返回它们的乘积。在这个函数中,我们使用三重嵌套的循环来计算乘积,并将结果存储在一个结果矩阵中。
阅读全文
相关推荐
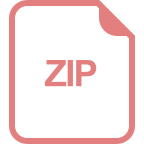
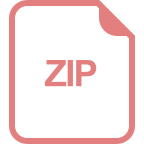















