1、实现如下功能:已知无向图的邻接表,判断该邻接表是否连通。 //1. 提示:函数可以写成如下形式BOOL judge_is_connected(adjList b[ ]) //2. 提示:(a)结构体自己定义;(b)定义提示1的函数
时间: 2023-06-12 21:06:47 浏览: 56
可以按照以下步骤实现该功能:
1. 定义一个结构体 `vertexNode` 存储每个顶点的信息,包括顶点编号和与该顶点相邻的顶点列表。
```c++
struct vertexNode {
int vertex; // 顶点编号
vector<int> adjacent; // 与该顶点相邻的顶点列表
};
```
2. 定义一个邻接表 `adjList` 存储整个图的信息,包括顶点总数和每个顶点的信息。
```c++
struct adjList {
int count; // 顶点总数
vector<vertexNode> vertices; // 每个顶点的信息
};
```
3. 实现 `judge_is_connected(adjList b[])` 函数,判断邻接表是否连通。
```c++
bool judge_is_connected(adjList b[]) {
int n = b[0].count; // 获取顶点总数
vector<bool> visited(n, false); // 初始化所有顶点都未被访问
// 从第一个顶点开始遍历
dfs(b, visited, 0);
// 判断是否所有顶点都被访问过,如果有未被访问的顶点,则图不连通
for (int i = 0; i < n; i++) {
if (!visited[i]) {
return false;
}
}
return true;
}
```
4. 实现深度优先搜索函数 `dfs`,遍历图并标记已访问的顶点。
```c++
void dfs(adjList b[], vector<bool>& visited, int v) {
visited[v] = true; // 标记当前顶点已被访问
// 遍历当前顶点的相邻顶点
for (int i = 0; i < b[v].vertices.size(); i++) {
int w = b[v].vertices[i].vertex; // 获取相邻顶点的编号
if (!visited[w]) {
dfs(b, visited, w); // 递归访问相邻顶点
}
}
}
```
完整代码如下:
```c++
#include <iostream>
#include <vector>
using namespace std;
struct vertexNode {
int vertex; // 顶点编号
vector<int> adjacent; // 与该顶点相邻的顶点列表
};
struct adjList {
int count; // 顶点总数
vector<vertexNode> vertices; // 每个顶点的信息
};
bool judge_is_connected(adjList b[]) {
int n = b[0].count; // 获取顶点总数
vector<bool> visited(n, false); // 初始化所有顶点都未被访问
// 从第一个顶点开始遍历
dfs(b, visited, 0);
// 判断是否所有顶点都被访问过,如果有未被访问的顶点,则图不连通
for (int i = 0; i < n; i++) {
if (!visited[i]) {
return false;
}
}
return true;
}
void dfs(adjList b[], vector<bool>& visited, int v) {
visited[v] = true; // 标记当前顶点已被访问
// 遍历当前顶点的相邻顶点
for (int i = 0; i < b[v].vertices.size(); i++) {
int w = b[v].vertices[i].vertex; // 获取相邻顶点的编号
if (!visited[w]) {
dfs(b, visited, w); // 递归访问相邻顶点
}
}
}
int main() {
// 构建一个连通的无向图
adjList b[1];
b[0].count = 4;
vector<vertexNode> vertices(4);
vertices[0].vertex = 0;
vertices[0].adjacent = {1, 2};
vertices[1].vertex = 1;
vertices[1].adjacent = {0, 2, 3};
vertices[2].vertex = 2;
vertices[2].adjacent = {0, 1};
vertices[3].vertex = 3;
vertices[3].adjacent = {1};
b[0].vertices = vertices;
// 判断该邻接表是否连通
if (judge_is_connected(b)) {
cout << "该邻接表表示的无向图是连通的" << endl;
} else {
cout << "该邻接表表示的无向图是不连通的" << endl;
}
return 0;
}
```
相关推荐
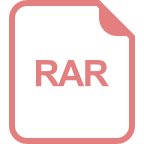
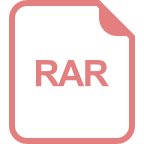














