已知无向图的邻接表,判断该邻接表是否连通的c语言代码
时间: 2023-06-11 16:08:31 浏览: 50
可以使用深度优先搜索(DFS)或广度优先搜索(BFS)来判断邻接表是否连通。以下是使用DFS的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#define MAX_VERTEX_NUM 100
typedef struct ArcNode {
int adjvex; // 相邻结点的下标
struct ArcNode *nextarc; // 指向下一个相邻结点的指针
} ArcNode;
typedef struct VertexNode {
int data; // 顶点的数据
ArcNode *firstarc; // 指向第一个相邻结点的指针
} VertexNode;
typedef struct Graph {
VertexNode vertex[MAX_VERTEX_NUM]; // 图的顶点数组
int vexnum, arcnum; // 顶点数和边数
} Graph;
int visited[MAX_VERTEX_NUM]; // 标记顶点是否被访问过
void DFS(Graph *G, int v) {
visited[v] = 1; // 标记顶点v已被访问
ArcNode *p = G->vertex[v].firstarc;
while (p != NULL) {
int w = p->adjvex; // w是v的相邻结点
if (!visited[w]) { // 如果w未被访问,则对w进行DFS
DFS(G, w);
}
p = p->nextarc;
}
}
int main() {
// 读入邻接表
Graph G;
scanf("%d%d", &G.vexnum, &G.arcnum);
for (int i = 0; i < G.vexnum; i++) {
G.vertex[i].data = i; // 顶点数据为下标
G.vertex[i].firstarc = NULL; // 初始时没有相邻结点
}
for (int i = 0; i < G.arcnum; i++) {
int u, v;
scanf("%d%d", &u, &v);
// 在u和v之间建立一条边
ArcNode *p = (ArcNode *) malloc(sizeof(ArcNode));
p->adjvex = v;
p->nextarc = G.vertex[u].firstarc;
G.vertex[u].firstarc = p;
// 由于是无向图,还需要在v和u之间建立一条边
p = (ArcNode *) malloc(sizeof(ArcNode));
p->adjvex = u;
p->nextarc = G.vertex[v].firstarc;
G.vertex[v].firstarc = p;
}
// DFS遍历图,并判断是否连通
for (int i = 0; i < G.vexnum; i++) {
visited[i] = 0; // 初始化所有顶点未被访问
}
DFS(&G, 0); // 从任意一个顶点开始遍历
for (int i = 0; i < G.vexnum; i++) {
if (!visited[i]) {
printf("该图不连通\n");
return 0;
}
}
printf("该图连通\n");
return 0;
}
```
该程序使用邻接表存储图,并对每个顶点进行DFS遍历。如果遍历过程中发现有未被访问的顶点,则图不是连通的。
相关推荐
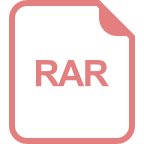
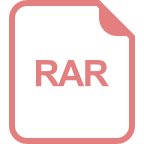














