用c++写一个游乐场管理系统,基本要求,能从文件里读出游乐场管理信息,并能将管理信息保存到文件。游乐场信息包括:游乐设施编号、游乐设备名称、管理人员、消费金额、消费日期。功能要求:增加和删除游乐场的消费记录,查询游乐场某个游乐项目某日的收入总额,查询游乐场某日的总收入,查询所有游乐设备的收入情况,并能根据某时段和某日的游乐设备收入高低排序,提供一个程序结构
时间: 2024-02-22 10:58:53 浏览: 177
好的,我可以帮你解答这个问题。下面是一个简单的游乐场管理系统的程序结构,你可以根据这个结构进行代码实现:
```c++
#include <iostream>
#include <fstream>
#include <string>
#include <vector>
#include <algorithm>
#include <ctime>
using namespace std;
// 游乐设施结构体定义
struct Facility {
string id; // 游乐设施编号
string name; // 游乐设备名称
string manager; // 管理人员
double price; // 消费金额
time_t date; // 消费日期
};
// 游乐场管理系统类定义
class AmusementPark {
public:
AmusementPark(string filename); // 构造函数,从文件中读取游乐场信息
void saveToFile(string filename); // 将游乐场信息保存到文件
void addRecord(Facility record); // 增加游乐场消费记录
void deleteRecord(int index); // 删除游乐场消费记录
double getIncomeByFacilityAndDate(string facilityId, time_t date); // 查询某个游乐项目某日的收入总额
double getTotalIncomeByDate(time_t date); // 查询某日的总收入
vector<pair<string, double>> getFacilityRevenueByPeriod(time_t start, time_t end); // 查询某时段游乐设备收入高低排序
private:
vector<Facility> records; // 游乐场消费记录
};
// 构造函数,从文件中读取游乐场信息
AmusementPark::AmusementPark(string filename) {
ifstream fin(filename);
string line;
while (getline(fin, line)) {
Facility record;
// 从文件中读取游乐场信息
// 将读取到的信息存入record中
records.push_back(record);
}
}
// 将游乐场信息保存到文件
void AmusementPark::saveToFile(string filename) {
ofstream fout(filename);
for (Facility record : records) {
// 将游乐场信息写入文件
}
fout.close();
}
// 增加游乐场消费记录
void AmusementPark::addRecord(Facility record) {
records.push_back(record);
}
// 删除游乐场消费记录
void AmusementPark::deleteRecord(int index) {
records.erase(records.begin() + index);
}
// 查询某个游乐项目某日的收入总额
double AmusementPark::getIncomeByFacilityAndDate(string facilityId, time_t date) {
double income = 0;
for (Facility record : records) {
if (record.id == facilityId && record.date == date) {
income += record.price;
}
}
return income;
}
// 查询某日的总收入
double AmusementPark::getTotalIncomeByDate(time_t date) {
double income = 0;
for (Facility record : records) {
if (record.date == date) {
income += record.price;
}
}
return income;
}
// 查询某时段游乐设备收入高低排序
vector<pair<string, double>> AmusementPark::getFacilityRevenueByPeriod(time_t start, time_t end) {
// 统计每个游乐设备的收入
vector<pair<string, double>> revenue;
for (Facility record : records) {
if (record.date >= start && record.date <= end) {
bool found = false;
for (int i = 0; i < revenue.size(); i++) {
if (revenue[i].first == record.name) {
revenue[i].second += record.price;
found = true;
break;
}
}
if (!found) {
revenue.push_back(make_pair(record.name, record.price));
}
}
}
// 按收入从高到低排序
sort(revenue.begin(), revenue.end(), [](const pair<string, double>& a, const pair<string, double>& b) {
return a.second > b.second;
});
return revenue;
}
int main() {
// 从文件中读取游乐场信息
AmusementPark park("amusement_park.txt");
// 增加游乐场消费记录
Facility record;
park.addRecord(record);
// 删除游乐场消费记录
park.deleteRecord(0);
// 查询某个游乐项目某日的收入总额
double income = park.getIncomeByFacilityAndDate("001", time(nullptr));
// 查询某日的总收入
income = park.getTotalIncomeByDate(time(nullptr));
// 查询某时段游乐设备收入高低排序
vector<pair<string, double>> revenue = park.getFacilityRevenueByPeriod(time(nullptr), time(nullptr));
// 将游乐场信息保存到文件
park.saveToFile("amusement_park.txt");
return 0;
}
```
上面的代码是一个基本的游乐场管理系统的程序结构,其中包含了一些基本功能,例如从文件中读取游乐场信息、将游乐场信息保存到文件、增加游乐场消费记录、删除游乐场消费记录、查询某个游乐项目某日的收入总额、查询某日的总收入、查询某时段游乐设备收入高低排序等。你可以根据这个程序结构进行代码实现,具体实现细节需要根据实际情况进行调整。
阅读全文
相关推荐
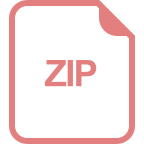
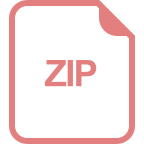
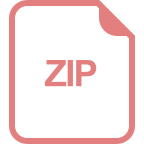
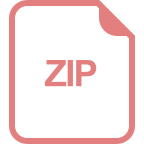
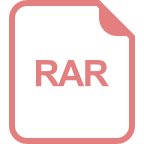
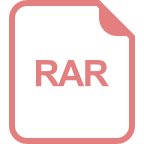
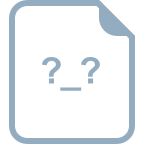
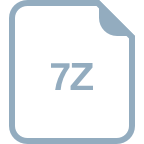
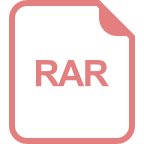
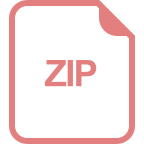
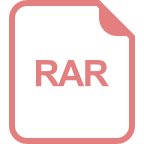
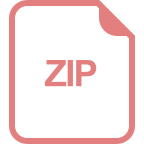
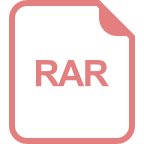
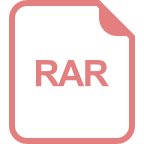
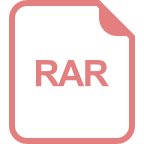