c语言实现地图着色问题
时间: 2023-08-17 11:27:41 浏览: 180
地图着色问题是一个著名的 NP 完全问题,其本质是给定一个地图,要求给地图中的每一个区域染上不同的颜色,使得相邻的区域颜色不同。在 C 语言中,可以使用图论算法来解决这个问题。
一种常见的算法是使用贪心策略,对地图的每个区域进行染色。具体步骤如下:
1. 初始化颜色集合,包括所有可能用到的颜色。
2. 对地图中的每个区域进行遍历。
3. 对于当前遍历到的区域,遍历其相邻的区域。
4. 对于每个相邻的区域,如果其已经被染色,则从颜色集合中删除该颜色。
5. 将剩余的颜色中最小的颜色赋给当前区域。
下面是一个简单的实现示例:
```c
#include <stdio.h>
#include <stdbool.h>
#define MAX_REGIONS 100
#define MAX_COLORS 10
int map[MAX_REGIONS][MAX_REGIONS]; // 地图
int colors[MAX_REGIONS]; // 每个区域的颜色
int n, m; // 区域数目和颜色数目
// 判断两个区域是否相邻
bool is_adjacent(int r1, int r2) {
// TODO: 实现判断逻辑
}
// 获取相邻区域的颜色
void get_adjacent_colors(int region, int adjacent_colors[]) {
// TODO: 实现获取逻辑
}
// 染色算法
void color_map() {
int i, j, k;
int adjacent_colors[MAX_COLORS];
// 遍历每个区域
for (i = 0; i < n; i++) {
// 获取相邻区域的颜色
get_adjacent_colors(i, adjacent_colors);
// 从颜色集合中删除相邻区域已经使用的颜色
for (j = 0; j < n; j++) {
if (map[i][j] && colors[j] != -1) {
for (k = 0; k < m; k++) {
if (adjacent_colors[k] == colors[j]) {
adjacent_colors[k] = -1;
}
}
}
}
// 选择最小的可用颜色
for (j = 0; j < m; j++) {
if (adjacent_colors[j] != -1) {
colors[i] = adjacent_colors[j];
break;
}
}
}
}
// 主函数
int main() {
int i, j;
// 读入地图
printf("Enter number of regions: ");
scanf("%d", &n);
printf("Enter adjacency matrix:\n");
for (i = 0; i < n; i++) {
for (j = 0; j < n; j++) {
scanf("%d", &map[i][j]);
}
}
// 初始化颜色
printf("Enter number of colors: ");
scanf("%d", &m);
for (i = 0; i < n; i++) {
colors[i] = -1;
}
// 染色
color_map();
// 输出结果
printf("Coloring:\n");
for (i = 0; i < n; i++) {
printf("Region %d: Color %d\n", i, colors[i]);
}
return 0;
}
```
需要注意的是,这只是一个简单的实现示例,实际应用中可能需要更复杂的算法来解决更为复杂的地图着色问题。
阅读全文
相关推荐
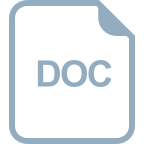
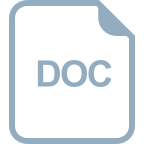
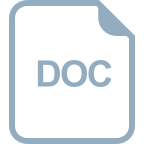
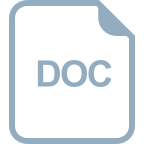

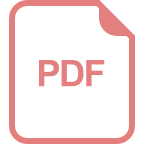
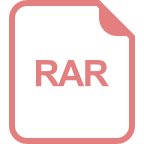
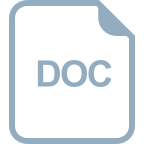
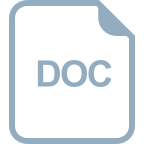
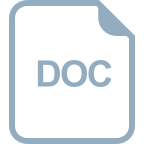
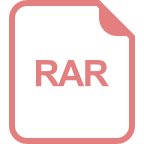
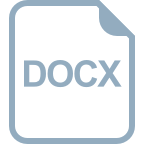
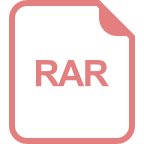
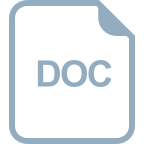
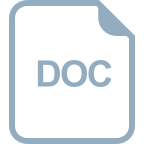