优化这段代码:#include <stdio.h> #include <stdlib.h> typedef struct node { int data; struct node* next; } Node; Node* create(int data) { Node* node = (Node*)malloc(sizeof(Node)); node->data = data; node->next = NULL; return node; } void print(Node* head) { while (head->next) { printf("%d->", head->next->data); head = head->next; } printf("\n"); } void destroy(Node* head) { while (head) { Node* temp = head->next; free(head); head = temp; } } void insert(Node* head, int data) { Node* node = create(data); Node* cur = head; while (cur->next && cur->next->data < data) { cur = cur->next; } node->next = cur->next; cur->next = node; } int main() { Node* p= create(-1); Node* n= create(-1); int data; while (scanf("%d", &data) != EOF) { if (data > 0) { insert(p, data); } else { insert(n, data); } } print(p); print(n); destroy(p); destroy(n); return 0; }
时间: 2024-03-05 20:49:48 浏览: 68
下面是优化后的代码:
```c
#include <stdio.h>
#include <stdlib.h>
typedef struct node {
int data;
struct node* next;
} Node;
Node* create(int data) {
Node* node = malloc(sizeof(Node));
node->data = data;
node->next = NULL;
return node;
}
void print(Node* head) {
for (Node* cur = head->next; cur != NULL; cur = cur->next) {
printf("%d->", cur->data);
}
printf("\n");
}
void destroy(Node* head) {
Node* cur = head;
while (cur != NULL) {
Node* next = cur->next;
free(cur);
cur = next;
}
}
void insert(Node* head, int data) {
Node* node = create(data);
Node* cur = head;
while (cur->next != NULL && cur->next->data < data) {
cur = cur->next;
}
node->next = cur->next;
cur->next = node;
}
int main() {
Node* positive = create(-1);
Node* negative = create(-1);
int data;
while (scanf("%d", &data) != EOF) {
if (data > 0) {
insert(positive, data);
} else {
insert(negative, data);
}
}
print(positive);
print(negative);
destroy(positive);
destroy(negative);
return 0;
}
```
优化的主要方式包括:
1. 将 `malloc` 的返回值直接赋值给指针变量,不需要强制类型转换。
2. 在 `print` 和 `destroy` 函数中使用 `for` 循环而不是 `while` 循环,这样可以避免在循环体外初始化循环变量。
3. 在 `destroy` 函数中,使用 `next` 指针保存当前结点的下一个结点,避免在释放当前结点后访问已经释放的内存。
4. 在 `insert` 函数中,将判断 `cur->next` 是否为空和 `cur->next->data` 是否小于 `data` 的逻辑合并成一个条件判断,避免重复访问 `cur->next`。
5. 将 `p` 更改为 `positive`,`n` 更改为 `negative`,使变量名更加清晰易懂。
阅读全文
相关推荐
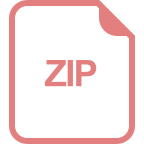
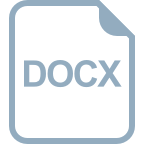
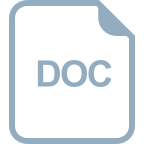
















