model = torch.load(weight_path)的weight_path应当是什么格式
时间: 2024-02-03 14:13:57 浏览: 25
`weight_path` 应该是一个包含模型参数的文件路径,通常是以 `.pt`、`.pth`、`.pkl`、`.ckpt` 等格式保存的。具体格式取决于你使用的框架和代码实现方式。在 PyTorch 中,我们可以使用 `torch.save()` 函数将模型保存为 `.pt` 或 `.pth` 格式的文件,然后使用 `torch.load()` 函数加载模型参数。例如:
```
# 保存模型
torch.save(model.state_dict(), "model.pt")
# 加载模型
model.load_state_dict(torch.load("model.pt"))
```
相关问题
model = resnet50(num_classes=2) # load model weights model_weight_path = "./resNet50.pth" model.load_state_dict(torch.load(model_weight_path,map_location='cpu')) model.eval()
这段代码是在 PyTorch 中加载预训练的 ResNet50 模型,并载入预训练的模型权重。具体来说,它包括以下步骤:
1. `resnet50(num_classes=2)`:创建一个 ResNet50 模型实例,并设置 `num_classes=2` 表示输出类别数为 2,因为该模型是用于二分类任务。
2. `model_weight_path = "./resNet50.pth"`:设置模型权重文件的路径,即预训练的 ResNet50 模型的权重文件。
3. `model.load_state_dict(torch.load(model_weight_path,map_location='cpu'))`:使用 PyTorch 的 `load_state_dict()` 函数加载预训练的模型权重。其中 `torch.load()` 函数将模型权重文件加载到内存中,并使用 `load_state_dict()` 函数将其赋值给模型实例。
4. `model.eval()`:将模型设置为评估模式,即不进行梯度计算和反向传播,只进行前向传播计算输出结果。
这些步骤将预训练的 ResNet50 模型加载到内存中,并准备好进行预测。可以使用该模型对图像进行分类等机器学习任务。
为以下每句代码做注释:device = torch.device("cuda:0" if torch.cuda.is_available() else "cpu") data_transform = transforms.Compose( [transforms.Resize(256), transforms.CenterCrop(224), transforms.ToTensor(), transforms.Normalize([0.485, 0.456, 0.406], [0.229, 0.224, 0.225])]) img = Image.open("./huanglongbing.JPG") plt.imshow(img) img = data_transform(img) img = torch.unsqueeze(img, dim=0) try: json_file = open('./class_indices.json', 'r') class_indict = json.load(json_file) except Exception as e: print(e) exit(-1) model = resnet152(num_classes=38) model_weight_path = "./resNet152.pth" model.load_state_dict(torch.load(model_weight_path, map_location=device)) model.eval() with torch.no_grad(): output = torch.squeeze(model(img)) predict = torch.softmax(output, dim=0) predict_cla = torch.argmax(predict).numpy() print(class_indict[str(predict_cla)], predict[predict_cla].numpy()) plt.show()
# 设备选择:如果有可用的cuda设备,则使用cuda:0,否则使用cpu
device = torch.device("cuda:0" if torch.cuda.is_available() else "cpu")
# 数据变换操作,包括图像大小调整、中心裁剪、转换为张量、归一化等
data_transform = transforms.Compose(
[transforms.Resize(256),
transforms.CenterCrop(224),
transforms.ToTensor(),
transforms.Normalize([0.485, 0.456, 0.406], [0.229, 0.224, 0.225])]
)
# 打开图像文件,显示图像
img = Image.open("./huanglongbing.JPG")
plt.imshow(img)
# 对图像进行数据变换
img = data_transform(img)
img = torch.unsqueeze(img, dim=0)
# 读取类别标签与索引的对应关系
try:
json_file = open('./class_indices.json', 'r')
class_indict = json.load(json_file)
except Exception as e:
print(e)
exit(-1)
# 加载预训练的resnet152模型,并载入预训练权重
model = resnet152(num_classes=38)
model_weight_path = "./resNet152.pth"
model.load_state_dict(torch.load(model_weight_path, map_location=device))
model.eval()
# 使用载入的模型进行推理,并输出预测结果
with torch.no_grad():
output = torch.squeeze(model(img))
predict = torch.softmax(output, dim=0)
predict_cla = torch.argmax(predict).numpy()
print(class_indict[str(predict_cla)], predict[predict_cla].numpy())
plt.show()
相关推荐
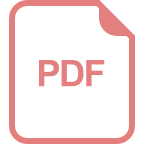
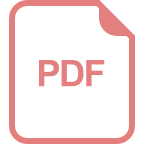













