代码解读: cnn = model.CNN(img_size=config.img_size, num_class=num_class).to(device) cnn.load_state_dict( torch.load(os.path.join(config.model_path, config.model_name)) ) finalconv_name = 'conv' # hook feature_blobs = [] def hook_feature(module, input, output): feature_blobs.append(output.cpu().data.numpy()) cnn._modules.get(finalconv_name).register_forward_hook(hook_feature) params = list(cnn.parameters()) # get weight only from the last layer(linear) weight_softmax = np.squeeze(params[-2].cpu().data.numpy())
时间: 2024-04-29 14:19:29 浏览: 147
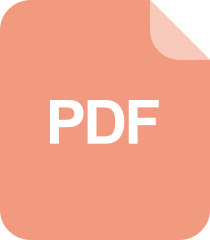
CNN_book中文版(魏秀参)

这段代码主要是加载一个预训练的卷积神经网络模型,并获取模型的最后一层的权重。
首先,代码通过调用 `model.CNN` 函数创建一个名为 `cnn` 的卷积神经网络模型,并将模型移动到指定的设备上。其输入图像的尺寸是 `config.img_size`,输出类别数目为 `num_class`。
接下来,代码通过调用 `torch.load` 函数加载预训练模型的参数,并使用 `load_state_dict` 函数将参数加载到 `cnn` 模型中。
然后,代码定义了一个名为 `finalconv_name` 的字符串变量,用于指定模型中最后一层的名称。通过使用 `register_forward_hook` 函数,代码将 `hook_feature` 函数注册到模型的最后一层中,以便在前向传递期间获取该层的输出。
最后,代码获取模型的所有参数,并从最后一层的权重中提取出一个名为 `weight_softmax` 的 numpy 数组,该数组将用于后续的可视化操作。具体来说,它从 `params` 列表中获取最后一层的参数,并使用 `np.squeeze` 函数将其转换为一个一维数组。
阅读全文
相关推荐
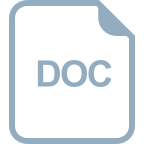
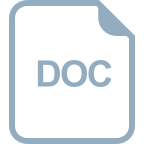
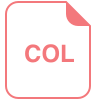
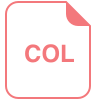
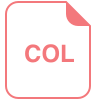
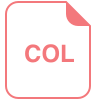
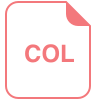
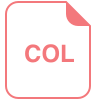
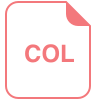
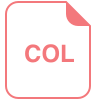
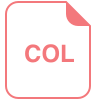
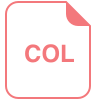
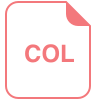
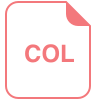
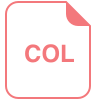
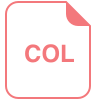

