class CNNmodel(nn.Module): def __init__(self): super(CNNmodel, self).__init__() self.conv1d = nn.Conv1d(1, 64, kernel_size=2) self.relu = nn.ReLU(inplace=True) self.Linear1 = nn.Linear(64*75, 50) self.Linear2 = nn.Linear(50, 1) def forward(self, x): x = self.conv1d(x) x = self.relu(x) x = x.view(-1) x = self.Linear1(x) x = self.relu(x) x = self.Linear2(x) return x报错
时间: 2023-11-07 07:04:40 浏览: 135
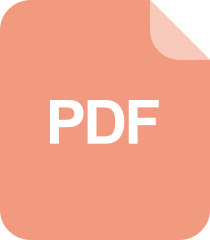
浅析PyTorch中nn.Module的使用

这段代码存在一些问题,导致运行时报错。以下是可能出现的问题及解决方法:
1. `x = x.view(-1)`这一行应该改为`x = x.view(-1, 64*75)`,因为在前面`self.conv1d`的输出通道数为64,而输出的feature map长度为75,需要将其展平后输入全连接层。
2. `self.Linear2 = nn.Linear(50, 1)`中的1代表输出的类别数,如果是二分类问题,应该改为`self.Linear2 = nn.Linear(50, 2)`,并在模型的输出处加上softmax激活函数。
修改后的代码如下:
```
class CNNmodel(nn.Module):
def __init__(self):
super(CNNmodel, self).__init__()
self.conv1d = nn.Conv1d(1, 64, kernel_size=2)
self.relu = nn.ReLU(inplace=True)
self.Linear1 = nn.Linear(64*75, 50)
self.Linear2 = nn.Linear(50, 2)
def forward(self, x):
x = self.conv1d(x)
x = self.relu(x)
x = x.view(-1, 64*75)
x = self.Linear1(x)
x = self.relu(x)
x = self.Linear2(x)
x = F.softmax(x, dim=1)
return x
```
阅读全文
相关推荐
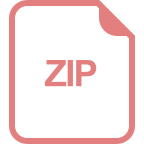
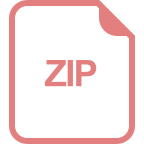
















