设计程序复制一个进程,父进程读取一段源程序的内容,删除其中的注释后通过管道发送给子进程,并呈现在屏幕上。程序中用管道实现了文件的传输,另外删除源代码中的注释也是一个挺实用的功能,这里的注释特指/,/与它们之间的内容
时间: 2024-02-20 12:00:35 浏览: 29
好的,这是一个关于进程、管道和文件操作的问题。我来一步步解答:
1. 首先,我们需要用 fork() 函数来创建一个子进程。子进程将会负责接收父进程传来的源程序内容,并删除其中的注释。
2. 接下来,父进程需要读取源程序的内容。我们可以使用 fopen() 函数打开源程序文件,然后使用 fgets() 函数逐行读取该文件的内容。读取的内容可以暂时存储在一个缓冲区中,等待后续处理。
3. 父进程需要删除注释。我们可以编写一个函数来实现该功能。该函数可以扫描缓冲区中的每一行,找到注释的起始位置(即第一个 /),然后找到注释的结束位置(即第二个 /),并将它们之间的内容删除。
4. 接下来,父进程需要使用管道将处理后的源程序内容传给子进程。我们可以使用 pipe() 函数创建一个管道,然后使用 write() 函数将处理后的内容写入管道中。
5. 子进程需要接收管道中的内容,并将其呈现在屏幕上。我们可以使用 read() 函数从管道中读取内容,然后使用 printf() 函数将其输出到屏幕上。
总体来说,这个程序需要使用多个函数和系统调用来实现。你可以参考下面的代码框架来编写程序:
```c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <string.h>
#define BUFSIZE 1024
void remove_comments(char *buf);
void child_process(int fd);
void parent_process(int fd);
int main(int argc, char **argv)
{
FILE *fp;
char buf[BUFSIZE];
pid_t pid;
int fd[2];
if (argc != 2) {
fprintf(stderr, "Usage: %s <filename>\n", argv[0]);
exit(1);
}
if (pipe(fd) == -1) {
perror("pipe");
exit(1);
}
pid = fork();
if (pid == -1) {
perror("fork");
exit(1);
}
if (pid == 0) {
// child process
close(fd[1]);
child_process(fd[0]);
} else {
// parent process
close(fd[0]);
fp = fopen(argv[1], "r");
if (fp == NULL) {
perror("fopen");
exit(1);
}
while (fgets(buf, BUFSIZE, fp) != NULL) {
remove_comments(buf);
write(fd[1], buf, strlen(buf));
}
fclose(fp);
parent_process(fd[1]);
}
return 0;
}
void remove_comments(char *buf)
{
// TODO: remove comments in buf
}
void child_process(int fd)
{
char buf[BUFSIZE];
ssize_t nbytes;
while ((nbytes = read(fd, buf, BUFSIZE)) > 0) {
write(STDOUT_FILENO, buf, nbytes);
}
}
void parent_process(int fd)
{
// TODO: cleanup
}
```
这个代码框架只是一个简单的示例,你需要自己编写 remove_comments() 函数来删除注释,在 parent_process() 函数中添加必要的清理代码等等。
相关推荐
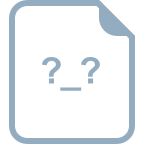
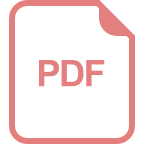














