verilog实现hdlc
时间: 2023-10-04 09:12:29 浏览: 113
HDLC是一种数据链路层协议,用于在不可靠的物理介质上可靠地传输数据。在Verilog中实现HDLC需要进行以下步骤:
1. 定义接口:首先需要定义接口,包括数据输入和输出端口、时钟和复位信号等。
2. 帧同步:HDLC帧的开始和结束需要使用特定的比特模式进行同步。在Verilog中,可以使用状态机实现帧同步功能。
3. 帧解析:读取接收到的数据,并进行帧解析。帧解析需要实现HDLC协议的功能,包括地址识别、帧类型判断、CRC检验等。
4. 帧生成:生成要发送的HDLC帧。生成帧需要实现HDLC协议的功能,包括地址设置、帧类型设置、CRC计算等。
5. 测试验证:完成以上步骤后,需要进行测试验证,验证HDLC协议的实现是否正确。
总之,实现Verilog中的HDLC需要综合考虑帧同步、帧解析、帧生成等多个方面,需要充分理解HDLC协议的原理和功能。
相关问题
verilog实现hdlc代码
下面是一份简单的Verilog HDLC代码示例,实现了基本的帧同步、帧解析和帧生成功能:
```verilog
module hdlc (
input clk,
input rstn,
input din,
output dout
);
// 定义状态机状态
parameter IDLE = 2'b00;
parameter FLAG = 2'b01;
parameter ADDR = 2'b10;
parameter CTL = 2'b11;
// 定义帧类型
parameter TYPE_DATA = 4'h0;
parameter TYPE_ACK = 4'h1;
// 定义帧控制字段
parameter CTL_NORMAL = 4'h0;
parameter CTL_REJECT = 4'h1;
reg [1:0] state; // 状态机状态
reg [7:0] addr; // 地址
reg [3:0] type; // 帧类型
reg [3:0] ctl; // 帧控制字段
reg [31:0] crc; // CRC校验码
reg [7:0] data; // 数据
reg [7:0] tx_data; // 待发送的数据
reg [3:0] tx_count; // 发送计数器
reg [3:0] rx_count; // 接收计数器
reg [1:0] bit_count; // 当前位计数器
reg flag_detected; // 是否检测到帧同步标志
reg [2:0] crc_count; // CRC计算器
reg [2:0] crc_bit; // 当前CRC位计数器
reg [31:0] crc_reg; // CRC寄存器
reg [7:0] crc_poly; // CRC多项式
reg [7:0] crc_xor; // CRC异或值
reg [7:0] crc_out; // CRC输出值
reg tx_done; // 发送完成标志
reg rx_done; // 接收完成标志
reg [1:0] tx_state; // 发送状态
// 初始化状态机状态
initial begin
state = IDLE;
end
// 帧同步状态机
always @(posedge clk or negedge rstn) begin
if (~rstn) begin
state <= IDLE;
flag_detected <= 0;
bit_count <= 0;
rx_count <= 0;
crc_count <= 0;
crc_bit <= 0;
crc_reg <= 32'hFFFFFFFF;
crc_poly <= 8'h07;
crc_xor <= 8'hFF;
crc_out <= 8'h00;
end else begin
case (state)
IDLE: begin
if (din) begin
state <= FLAG;
flag_detected <= 1;
end
end
FLAG: begin
if (din) begin
if (flag_detected) begin
state <= ADDR;
addr <= din;
rx_count <= 1;
bit_count <= 0;
flag_detected <= 0;
end
end else begin
flag_detected <= 1;
end
end
ADDR: begin
if (din) begin
if (rx_count < 2) begin
addr <= {addr[6:0], din};
rx_count <= rx_count + 1;
end else if (rx_count == 2) begin
type <= din[3:0];
ctl <= din[7:4];
crc_count <= 0;
crc_bit <= 0;
crc_reg <= 32'hFFFFFFFF;
crc_out <= 8'h00;
state <= CTL;
rx_count <= rx_count + 1;
end
end else begin
state <= FLAG;
flag_detected <= 1;
end
end
CTL: begin
if (din) begin
if (crc_count < 4) begin
crc_reg <= crc_reg ^ {din, 24'h00} ^ (crc_reg & 8'hFF) ^ crc_poly;
crc_count <= crc_count + 1;
end else if (crc_count == 4) begin
crc_out <= ~crc_reg ^ crc_xor;
state <= FLAG;
flag_detected <= 1;
rx_done <= 1;
end
end else begin
data <= 0;
state <= FLAG;
flag_detected <= 1;
end
end
endcase
end
end
// 帧生成状态机
always @(posedge clk or negedge rstn) begin
if (~rstn) begin
state <= IDLE;
tx_count <= 0;
tx_done <= 0;
tx_state <= 2'b00;
end else begin
case (tx_state)
2'b00: begin
if (tx_count == 0) begin
tx_data <= 8'h7E;
tx_count <= 1;
end else begin
tx_state <= 2'b01;
tx_count <= 0;
end
end
2'b01: begin
if (tx_count == 0) begin
tx_data <= addr[7:0];
tx_count <= 1;
end else begin
tx_state <= 2'b10;
tx_count <= 0;
end
end
2'b10: begin
if (tx_count == 0) begin
tx_data <= addr[15:8];
tx_count <= 1;
end else begin
tx_state <= 2'b11;
tx_count <= 0;
end
end
2'b11: begin
if (tx_count == 0) begin
tx_data <= {ctl, type};
tx_count <= 1;
end else begin
tx_state <= 2'b100;
tx_count <= 0;
end
end
2'b100: begin
if (tx_count == 0) begin
tx_data <= data;
tx_count <= 1;
end else begin
tx_state <= 2'b101;
tx_count <= 0;
end
end
2'b101: begin
if (tx_count == 0) begin
tx_data <= crc_out;
tx_count <= 1;
end else begin
tx_state <= 2'b00;
tx_done <= 1;
tx_count <= 0;
end
end
endcase
end
end
// 数据接收和发送
assign dout = (tx_state == 2'b00) ? tx_data : 1'b0;
always @(posedge clk or negedge rstn) begin
if (~rstn) begin
data <= 0;
end else begin
if (rx_done) begin
// 数据接收完成
// 处理接收到的数据
// ...
rx_done <= 0;
end else begin
// 数据发送
if (tx_done) begin
// 发送完成
tx_done <= 0;
end else if (tx_state != 2'b00) begin
// 发送数据
data <= tx_data;
end
end
end
end
endmodule
```
上述代码仅作为示例,实际应用中需要根据具体需求进行修改和完善。
HDLC协议 verilog
根据提供的引用内容,HDLC协议是一种常用的通讯协议,而Verilog是一种硬件描述语言,用于设计和验证数字电路。因此,可以使用Verilog来实现HDLC协议。
下面是一个简单的Verilog代码示例,用于实现HDLC协议的基本功能:
```verilog
module hdlc_rx(
input clk,
input rst_n,
input rx_data,
input rx_valid,
output reg frame_error,
output reg [7:0] data_out,
output reg frame_valid
);
reg [7:0] shift_reg;
reg [7:0] data_reg;
reg [2:0] bit_count;
reg frame_sync;
parameter IDLE = 3'b000;
parameter FLAG = 3'b001;
parameter DATA = 3'b010;
parameter ESCAPE = 3'b011;
reg [2:0] state;
reg [2:0] next_state;
always @(posedge clk or negedge rst_n) begin
if (~rst_n) begin
state <= IDLE;
shift_reg <= 8'h00;
data_reg <= 8'h00;
bit_count <= 3'b000;
frame_sync <= 1'b0;
frame_error <= 1'b0;
frame_valid <= 1'b0;
end else begin
state <= next_state;
shift_reg <= {shift_reg[6:0], rx_data};
data_reg <= {data_reg[6:0], rx_data};
bit_count <= (state == DATA && bit_count == 3'b111) ? 3'b000 : bit_count + 1;
frame_sync <= (shift_reg == 8'h7e) ? 1'b1 : (shift_reg == 8'h7d && frame_sync) ? 1'b1 : (shift_reg != 8'h7d) ? 1'b0 : frame_sync;
end
end
always @(*) begin
case (state)
IDLE: begin
next_state = (rx_valid && rx_data == 8'h7e) ? FLAG : IDLE;
end
FLAG: begin
next_state = (rx_valid && rx_data == 8'h7e') ? FLAG : DATA;
end
DATA: begin
next_state = (rx_valid && rx_data == 8'h7e) ? FLAG : (rx_valid && rx_data == 8'h7d) ? ESCAPE : DATA;
end
ESCAPE: begin
next_state = (rx_valid) ? DATA : ESCAPE;
end
default: begin
next_state = IDLE;
end
endcase
end
always @(posedge clk or negedge rst_n) begin
if (~rst_n) begin
data_out <= 8'h00;
end else begin
case (state)
IDLE: begin
data_out <= 8'h00;
end
FLAG: begin
data_out <= 8'h00;
end
DATA: begin
data_out <= data_reg;
end
ESCAPE: begin
data_out <= rx_data ^ 8'h20;
end
default: begin
data_out <= 8'h00;
end
endcase
end
end
always @(posedge clk or negedge rst_n) begin
if (~rst_n) begin
frame_valid <= 1'b0;
end else begin
case (state)
IDLE: begin
frame_valid <= 1'b0;
end
FLAG: begin
frame_valid <= 1'b0;
end
DATA: begin
frame_valid <= (bit_count == 3'b000 && frame_sync) ? 1'b1 : 1'b0;
end
ESCAPE: begin
frame_valid <= 1'b0;
end
default: begin
frame_valid <= 1'b0;
end
endcase
end
end
always @(posedge clk or negedge rst_n) begin
if (~rst_n) begin
frame_error <= 1'b0;
end else begin
case (state)
IDLE: begin
frame_error <= 1'b0;
end
FLAG: begin
frame_error <= 1'b0;
end
DATA: begin
frame_error <= (bit_count == 3'b000 && !frame_sync) ? 1'b1 : 1'b0;
end
ESCAPE: begin
frame_error <= 1'b0;
end
default: begin
frame_error <= 1'b0;
end
endcase
end
end
endmodule
```
该代码实现了一个简单的HDLC接收器,包括帧同步、数据解码和错误检测等功能。需要注意的是,该代码仅用于演示Verilog实现HDLC协议的基本思路,实际应用中需要根据具体需求进行修改和优化。
相关推荐
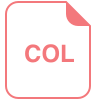
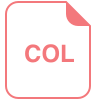
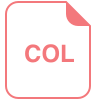
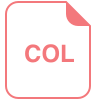
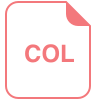







