QGraphicsItem图元怎么支持键盘输入焦点和按键事件
时间: 2024-01-24 13:09:21 浏览: 282
要支持键盘输入焦点和按键事件,QGraphicsItem需要继承自QGraphicsItem类,并且重写下列方法:
1. void keyPressEvent(QKeyEvent *event):重写keyPressEvent方法处理按键事件;
2. QVariant itemChange(GraphicsItemChange change, const QVariant &value):重写itemChange方法处理焦点事件。
在重写keyPressEvent方法时,需要注意:
1. QGraphicsItem没有默认的键盘焦点,因此需要使用setFlag()方法将QGraphicsItem的ItemIsFocusable标志设置为true,才能接收键盘事件;
2. 在keyPressEvent方法中,需要通过QKeyEvent的key()方法获取按下的键,并根据需要进行处理。
在重写itemChange方法时,需要注意:
1. 当焦点状态改变时,会触发GraphicsItemChange类型的change参数为QGraphicsItem::ItemFocusHasChanged的信号,可以在itemChange方法中重写处理该信号;
2. 在itemChange方法中,可以通过value参数获取焦点状态的改变,并根据需要进行处理。
举例来说,以下代码是QGraphicsItem的一个子类,在该类中实现了键盘输入焦点和按键事件的支持:
```c++
class MyItem : public QGraphicsItem
{
public:
MyItem(QGraphicsItem *parent = nullptr) : QGraphicsItem(parent)
{
setFlag(ItemIsFocusable, true);
}
QRectF boundingRect() const override
{
return QRectF(0, 0, 100, 100);
}
void paint(QPainter *painter, const QStyleOptionGraphicsItem *option, QWidget *widget = nullptr) override
{
painter->drawRect(boundingRect());
}
void keyPressEvent(QKeyEvent *event) override
{
switch (event->key()) {
case Qt::Key_Up:
setPos(pos() + QPointF(0, -10));
break;
case Qt::Key_Down:
setPos(pos() + QPointF(0, 10));
break;
case Qt::Key_Left:
setPos(pos() + QPointF(-10, 0));
break;
case Qt::Key_Right:
setPos(pos() + QPointF(10, 0));
break;
default:
break;
}
}
QVariant itemChange(GraphicsItemChange change, const QVariant &value) override
{
if (change == QGraphicsItem::ItemFocusHasChanged) {
if (value.toBool()) {
qDebug() << "Item has gained focus!";
} else {
qDebug() << "Item has lost focus!";
}
}
return QGraphicsItem::itemChange(change, value);
}
};
```
在上述代码中,MyItem类重写了QGraphicsItem的keyPressEvent和itemChange方法,实现了键盘输入焦点和按键事件的支持。具体地,该类在构造函数中将ItemIsFocusable标志设置为true,使其能够接受键盘焦点;在keyPressEvent方法中,根据按下的键进行移动;在itemChange方法中,处理焦点状态的改变。
阅读全文
相关推荐
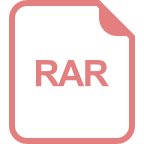
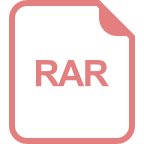
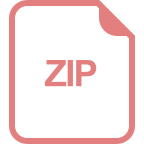

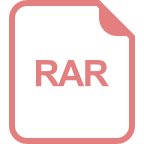
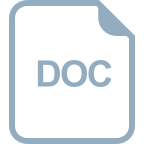











