1.整数运算示例,给定两个整数 a、b,计算两者之和。 2.浮点数运算示例,给定两个浮点数 x、y,计算两者之和。 3.将给定的值 a、b 转换为 bool 值,并输出两者的逻辑与运算结果。 4.字符串示例,格式化输出字符串堆积三角形。 5.通过声明和调用函数的方式,根据本金、年利率和年数,实现最终收益的计算。 6.定义一个类 Person,创建其对象,并调用对象方法。
时间: 2024-02-24 19:55:28 浏览: 92
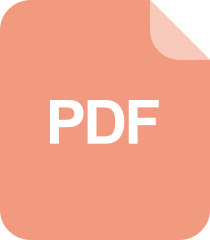
javascript 计算两个整数的百分比值
以下是六个问题的Python代码实现:
1. 整数运算示例,给定两个整数 a、b,计算两者之和。
```python
a = int(input("请输入第一个整数:"))
b = int(input("请输入第二个整数:"))
result = a + b
print("两数之和为:", result)
```
2. 浮点数运算示例,给定两个浮点数 x、y,计算两者之和。
```python
x = float(input("请输入第一个浮点数:"))
y = float(input("请输入第二个浮点数:"))
result = x + y
print("两数之和为:", result)
```
3. 将给定的值 a、b 转换为 bool 值,并输出两者的逻辑与运算结果。
```python
a = int(input("请输入第一个整数:"))
b = int(input("请输入第二个整数:"))
a_bool = bool(a)
b_bool = bool(b)
result = a_bool and b_bool
print("a和b的逻辑与结果为:", result)
```
4. 字符串示例,格式化输出字符串堆积三角形。
```python
n = int(input("请输入要输出的行数:"))
for i in range(1, n + 1):
print(" " * (n - i), "*" * (2 * i - 1))
```
5. 通过声明和调用函数的方式,根据本金、年利率和年数,实现最终收益的计算。
```python
def calculate_interest(principal, rate, years):
interest = principal * rate * years
return interest
principal = float(input("请输入本金:"))
rate = float(input("请输入年利率:"))
years = int(input("请输入年数:"))
interest = calculate_interest(principal, rate, years)
print("最终收益为:", interest)
```
6. 定义一个类 Person,创建其对象,并调用对象方法。
```python
class Person:
def __init__(self, name, age):
self.name = name
self.age = age
def say_hello(self):
print("大家好,我叫{},今年{}岁。".format(self.name, self.age))
person1 = Person("张三", 20)
person1.say_hello()
```
阅读全文
相关推荐
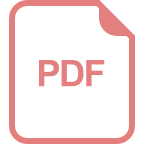
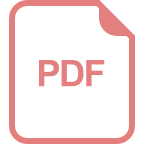
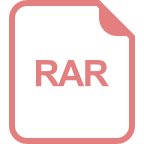
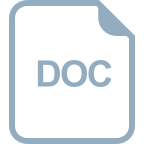
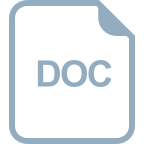
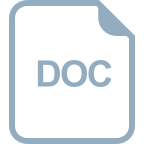
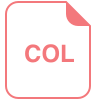










