请用c++和Matplotlibcpp库帮我实现matlab的contourc函数,并且不使用opencv
时间: 2024-03-21 20:42:47 浏览: 115
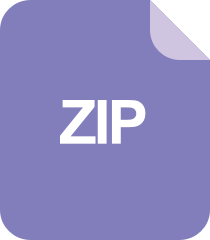
实现matlab矩阵计算的C++源程序
好的,以下是使用C++和Matplotlibcpp库实现类似于Matlab的contourc函数的示例代码:
```c++
#include <iostream>
#include <vector>
#include "matplotlibcpp.h"
namespace plt = matplotlibcpp;
// 定义数据结构表示一个点
struct Point {
double x, y;
Point(double x, double y) : x(x), y(y) {}
};
// 定义数据结构表示一个等高线
struct Contour {
double level;
std::vector<Point> points;
Contour(double level) : level(level) {}
};
// 定义函数计算二维数组的等高线
std::vector<Contour> contourc(std::vector<std::vector<double>> Z, std::vector<double> levels) {
// 定义变量
int n = Z.size(), m = Z[0].size();
std::vector<std::vector<bool>> visited(n, std::vector<bool>(m, false));
std::vector<Contour> contours;
// 遍历每个格点
for (int i = 0; i < n; i++) {
for (int j = 0; j < m; j++) {
// 如果该格点没有被访问过,且其高度在等高线高度级别中
if (!visited[i][j]) {
double z = Z[i][j];
for (double level : levels) {
if (z == level) {
// 如果该格点的高度等于当前高度级别,创建一个新的等高线
Contour contour(level);
std::vector<Point> &points = contour.points;
points.emplace_back(i, j);
visited[i][j] = true;
// 从该格点开始进行深度优先搜索,找到所有高度等于当前高度级别的格点
std::vector<std::pair<int, int>> stack = {{i, j}};
while (!stack.empty()) {
int x = stack.back().first, y = stack.back().second;
stack.pop_back();
if (visited[x][y]) {
continue;
}
visited[x][y] = true;
if (x > 0 && Z[x-1][y] == level) {
points.emplace_back(x-1, y);
stack.emplace_back(x-1, y);
}
if (x < n-1 && Z[x+1][y] == level) {
points.emplace_back(x+1, y);
stack.emplace_back(x+1, y);
}
if (y > 0 && Z[x][y-1] == level) {
points.emplace_back(x, y-1);
stack.emplace_back(x, y-1);
}
if (y < m-1 && Z[x][y+1] == level) {
points.emplace_back(x, y+1);
stack.emplace_back(x, y+1);
}
}
// 将等高线添加到结果中
contours.push_back(contour);
}
}
}
}
}
return contours;
}
int main() {
// 定义二维数组
std::vector<std::vector<double>> Z = {
{0.5, 0.1, 0.3, 0.2},
{0.4, 0.9, 0.7, 0.8},
{0.6, 0.2, 0.4, 0.1},
{0.1, 0.8, 0.6, 0.9}
};
// 计算等高线
std::vector<double> levels = {0.2, 0.4, 0.6, 0.8};
std::vector<Contour> contours = contourc(Z, levels);
// 绘制等高线
for (Contour &contour : contours) {
std::vector<double> x, y;
for (Point &point : contour.points) {
x.push_back(point.x);
y.push_back(point.y);
}
plt::plot(x, y);
}
plt::show();
return 0;
}
```
这段代码中,我们首先定义了Point和Contour两个数据结构,用于表示一个点和一个等高线。然后,我们定义了contourc函数,用于计算二维数组的等高线。函数中采用深度优先搜索的方法,从每个高度等于当前高度级别的格点出发,找到所有高度等于当前高度级别的格点,并将它们组成一个等高线。最后,我们使用Matplotlibcpp库中的plot()函数,绘制了所有等高线的图形。
阅读全文
相关推荐


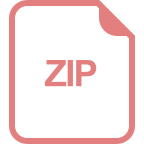
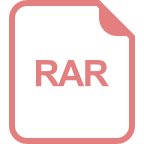
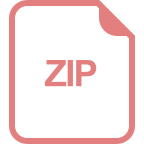
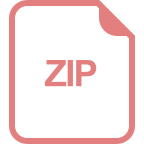


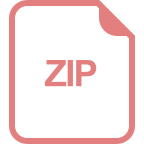
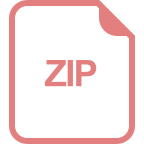
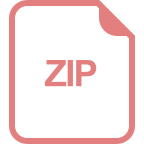
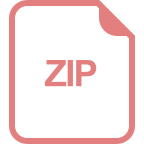
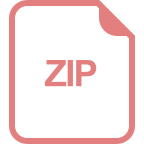
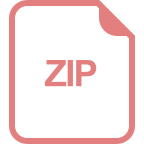
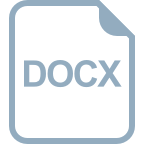

