c++ 实现F.interpolate
时间: 2023-08-08 21:10:11 浏览: 88
在 C++ 中实现 F.interpolate(PyTorch 中的函数)需要使用插值算法,可以使用线性插值或者双线性插值。
下面是一个简单的例子,使用双线性插值对输入的 2D Tensor 进行插值:
```c++
#include <torch/extension.h>
torch::Tensor interpolate(torch::Tensor input, torch::IntArrayRef output_size) {
auto batch_size = input.size(0);
auto input_height = input.size(2);
auto input_width = input.size(3);
auto output_height = output_size[0];
auto output_width = output_size[1];
auto output = torch::zeros({batch_size, input.size(1), output_height, output_width}, input.options());
for (int b = 0; b < batch_size; b++) {
for (int c = 0; c < input.size(1); c++) {
for (int h = 0; h < output_height; h++) {
for (int w = 0; w < output_width; w++) {
auto h_in = (float)(h + 0.5) * (float)input_height / (float)output_height - 0.5;
auto w_in = (float)(w + 0.5) * (float)input_width / (float)output_width - 0.5;
int h_low = floor(h_in);
int w_low = floor(w_in);
int h_high = h_low + 1;
int w_high = w_low + 1;
auto hl_ratio = h_in - h_low;
auto wl_ratio = w_in - w_low;
auto hh_ratio = 1 - hl_ratio;
auto wh_ratio = 1 - wl_ratio;
auto v1 = input[b][c][h_low][w_low];
auto v2 = input[b][c][h_low][w_high];
auto v3 = input[b][c][h_high][w_low];
auto v4 = input[b][c][h_high][w_high];
auto w1 = hh_ratio * wh_ratio;
auto w2 = hh_ratio * wl_ratio;
auto w3 = hl_ratio * wh_ratio;
auto w4 = hl_ratio * wl_ratio;
output[b][c][h][w] = v1 * w1 + v2 * w2 + v3 * w3 + v4 * w4;
}
}
}
}
return output;
}
PYBIND11_MODULE(TORCH_EXTENSION_NAME, m) {
m.def("interpolate", &interpolate, "F.interpolate");
}
```
这里使用了 PyTorch 的 C++ 扩展库。你可以将以上代码编译成 C++ 扩展模块,然后在 PyTorch 中使用 F.interpolate 函数。
相关推荐
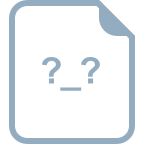














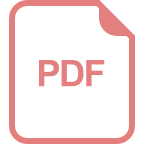