把这段代码改为在串行输入端依次读取字符int buffer_enqueue(uint8_t item) { if (!buffer_is_full()) { CharBuff[tail++] = item; tail %= UART_DMA_BUFF_LEN_MAX; return 1; } else { uart_disable(); // Disable UART when the buffer is full return 0; } } // Dequeue a character from the buffer int buffer_dequeue(uint8_t *item) { if (!buffer_is_empty()) { *item = CharBuff[head++]; head %= UART_DMA_BUFF_LEN_MAX; return 1; } else { return 0; } }
时间: 2024-03-14 14:45:19 浏览: 25
这段代码实现了一个先进先出(FIFO)缓冲区,用于存储 UART 接收到的字节数据。如果要将其改为在串行输入端依次读取字符,可以修改 buffer_dequeue() 函数,例如:
```c
// Read a character from the buffer
int buffer_read(char *ch) {
if (!buffer_is_empty()) {
*ch = CharBuff[head++];
head %= UART_DMA_BUFF_LEN_MAX;
return 1;
} else {
return 0;
}
}
```
这个函数每次从缓冲区中读取一个字符,并将其存储到指定的 ch 变量中。如果缓冲区为空,则返回 0。注意,返回值是实际读取的字符数,如果缓冲区中有多个字符,那么每次调用该函数只会读取一个字符。如果需要读取多个字符,可以多次调用该函数。
相关问题
把这段代码改为读取字符串int buffer_enqueue(uint8_t item) { if (!buffer_is_full()) { CharBuff[tail++] = item; tail %= UART_DMA_BUFF_LEN_MAX; return 1; } else { uart_disable(); // Disable UART when the buffer is full return 0; } } // Dequeue a character from the buffer int buffer_dequeue(uint8_t *item) { if (!buffer_is_empty()) { *item = CharBuff[head++]; head %= UART_DMA_BUFF_LEN_MAX; return 1; } else { return 0; } }
这段代码实现了一个先进先出(FIFO)缓冲区,用于存储 UART 接收到的字节数据。如果要将其改为读取字符串,可以稍微修改一下 buffer_dequeue() 函数,例如:
```c
// Dequeue a string from the buffer
int buffer_dequeue(char *str, size_t len) {
if (!buffer_is_empty()) {
size_t i;
for (i = 0; i < len && !buffer_is_empty(); i++) {
str[i] = CharBuff[head++];
head %= UART_DMA_BUFF_LEN_MAX;
}
str[i] = '\0';
return i;
} else {
return 0;
}
}
```
这个函数将从缓冲区中读取最多 len 个字节的数据,并存储到指定的字符串 str 中。如果缓冲区为空,则返回 0。注意,返回值是实际读取的字节数,如果缓冲区中不足 len 个字节,则只会读取缓冲区中的部分数据,并在字符串末尾添加一个 null 终止符。如果缓冲区中的数据长度超过了 len,那么多余的数据会被丢弃。
把这段代码改为先进先出改为先进先出int buffer_enqueue(uint8_t item) { if (!buffer_is_full()) { CharBuff[tail++] = item; tail %= UART_DMA_BUFF_LEN_MAX; return 1; } else { uart_disable(); // Disable UART when the buffer is full return 0; } } // Dequeue a character from the buffer int buffer_dequeue(uint8_t *item) { if (!buffer_is_empty()) { *item = CharBuff[head++]; head %= UART_DMA_BUFF_LEN_MAX; return 1; } else { return 0; } }
这段代码的作用是实现一个先进先出(FIFO)缓冲区,用于存储 UART 接收到的字节数据。具体来说,buffer_enqueue() 函数将一个字节添加到缓冲区的末尾,tail 指针指向下一个可写位置,如果缓冲区已满,则禁用 UART,并返回 0;buffer_dequeue() 函数从缓冲区的前端取出一个字节,并将其存储到指定的 item 变量中,head 指针指向下一个可读位置,如果缓冲区为空,则返回 0。这样,应用程序可以从缓冲区中读取数据,而不必担心数据的顺序。
相关推荐
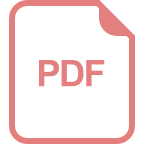
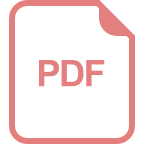
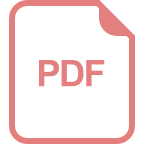












