键盘输入函数unity
时间: 2023-10-18 17:26:41 浏览: 129
在Unity中获取键盘输入可以使用以下代码:
```
void Update()
{
if (Input.GetKeyDown(KeyCode.Space))
{
// 在这里编写按下空格键时的逻辑
}
}
```
上述代码中,`Update()` 函数是在每一帧都会被调用的函数。当玩家按下空格键时,`Input.GetKeyDown()` 函数会返回 `true`,从而执行下面的逻辑。你可以根据自己的需要替换 `KeyCode.Space` 为其他按键的枚举值。
相关问题
unity检测键盘输入
在Unity中,可以使用Input类来检测键盘输入。以下是示例代码:
```
void Update() {
if (Input.GetKeyDown(KeyCode.Space)) {
Debug.Log("Space key was pressed.");
}
if (Input.GetKey(KeyCode.LeftShift)) {
Debug.Log("Left shift key is being held down.");
}
if (Input.GetKeyUp(KeyCode.Escape)) {
Debug.Log("Escape key was released.");
}
}
```
在上面的示例中,Update()函数每帧都会被调用。通过Input类的三个方法来检测键盘输入:
- GetKeyDown(KeyCode key): 检测某个键是否被按下,只在按下那一帧返回true。
- GetKey(KeyCode key): 检测某个键是否正在被按下,按下后持续返回true。
- GetKeyUp(KeyCode key): 检测某个键是否被释放,只在释放那一帧返回true。
unity inputSystem如何获取键盘输入?
Unity InputSystem 可以通过以下方式获取键盘输入:
1. 在 Unity 中创建一个 InputAction,并将其与键盘输入绑定。
2. 在 InputAction 中定义一个回调函数,用于处理键盘输入事件。
3. 在回调函数中,可以使用 InputSystem.GetDevice<Keyboard>() 方法获取键盘设备,并使用 Keyboard.current 读取当前键盘输入状态。
4. 通过读取键盘输入状态,可以获取按下的键、释放的键、持续按下的键等信息。
以下是示例代码:
```csharp
using UnityEngine;
using UnityEngine.InputSystem;
public class KeyboardInput : MonoBehaviour
{
private InputAction _keyboardAction;
private void Awake()
{
_keyboardAction = new InputAction(binding: "<Keyboard>/anyKey");
_keyboardAction.performed += OnKeyboardInput;
_keyboardAction.Enable();
}
private void OnKeyboardInput(InputAction.CallbackContext context)
{
var keyboard = InputSystem.GetDevice<Keyboard>();
if (keyboard == null)
{
return;
}
if (keyboard.anyKey.wasPressedThisFrame)
{
Debug.Log("A key was pressed.");
}
else if (keyboard.anyKey.wasReleasedThisFrame)
{
Debug.Log("A key was released.");
}
else if (keyboard.anyKey.isPressed)
{
Debug.Log("A key is being held down.");
}
}
}
```
阅读全文
相关推荐
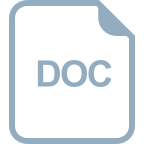
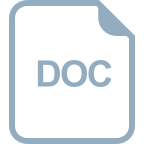
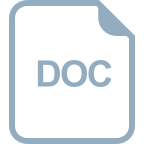
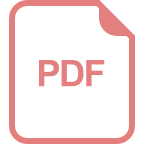
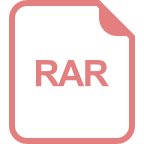
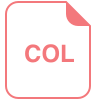
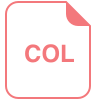









