用c语言编写一个程序,输入三个系数,求一元二次方程的解,要求输出所有可能的情况,包括复根。输入格式 三个实数a,b,c
时间: 2024-11-05 18:23:59 浏览: 19
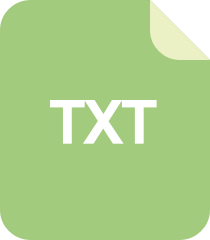
C语言编写的求一元二次方程的根(实根和复根)

为了编写一个C语言程序,解决这个问题,我们可以使用头文件`<complex.h>`来处理复数运算。以下是程序的基本结构:
```c
#include <stdio.h>
#include <complex.h>
// 函数声明
void calculateQuadraticRoots(double a, double b, double c);
int main() {
double a, b, c;
printf("请输入一元二次方程的系数 a, b, c:\n");
scanf("%lf %lf %lf", &a, &b, &c);
// 计算并打印结果
calculateQuadraticRoots(a, b, c);
return 0;
}
// 函数实现,用于计算和输出根
void calculateQuadraticRoots(double a, double b, double c) {
double discriminant = b * b - 4 * a * c;
if (discriminant > 0) { // 实数双根
double root1 = (-b + sqrt(discriminant)) / (2 * a);
double root2 = (-b - sqrt(discriminant)) / (2 * a);
printf("实数根:%.2f 和 %.2f\n", root1, root2);
} else if (discriminant == 0) { // 实数单根
double root = -b / (2 * a);
printf("实数根:%.2f\n", root);
} else { // 复数根
complex<double> realPart = -b / (2 * a), imaginaryPart = sqrt(-discriminant) / (2 * a);
complex<double> root1 = realPart + I * imaginaryPart;
complex<double> root2 = realPart - I * imaginaryPart;
printf("复数根:%.2f + %.2fi 和 %.2f - %.2fi\n", creal(root1), cimag(root1), creal(root2), cimag(root2));
}
}
```
这个程序首先从用户那里获取三个系数,然后通过`calculateQuadraticRoots`函数计算并打印出一元二次方程的所有可能解,包括实数双根、实数单根以及复数根。请注意,由于`complex.h`库的存在,该程序只能在支持复杂数的C环境中运行,如Linux或某些C++编译器。
阅读全文
相关推荐
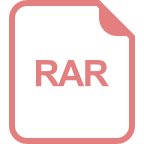
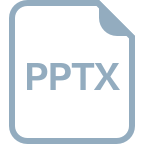

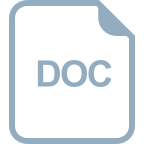
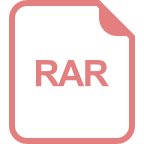
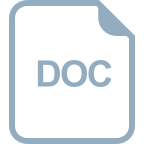
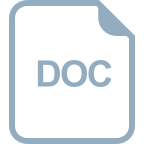

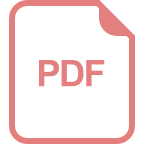
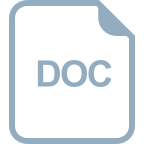
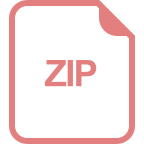
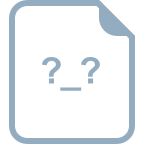

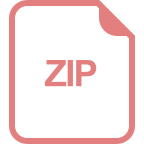