将列表[4,27,3,12,2,26,8,16,43,58]中的最后3个元素依次移到列表的首部,然后将列表降序排序后输出显示
时间: 2023-09-30 16:02:02 浏览: 31
可以使用以下代码来实现:
```python
lst = [4,27,3,12,2,26,8,16,43,58]
lst = lst[-3:] + lst[:-3] # 将最后3个元素移到首部
lst.sort(reverse=True) # 降序排序
print(lst) # 输出结果
```
输出结果为:`[58, 43, 27, 26, 16, 12, 8, 4, 3, 2]`
相关问题
输出一个 n 阶方阵,其元素排列方式为:从左上角开始按列顺序呈现出蛇形。如: 1 20 21 40 41 60 61 80 81 100 2 19 22 39 42 59 62 79 82 99 3 18 23 38 43 58 63 78 83 98 4 17 24 37 44 57 64 77 84 97 5 16 25 36 45 56 65 76 85 96 6 15 26 35 46 55 66 75 86 95 7 14 27 34 47 54 67 74 87 94 8 13 28 33 48 53 68 73 88 93 9 12 29 32 49 52 69 72 89 92 10 11 30 31 50 51 70 71 90 91 输入描述 每行一个正整数 n (n <= 120),表示 n 阶方阵。 输出描述 按列序排列的蛇形矩阵,每个方阵之间空一行。每个矩阵元素间一个空格,包含最后一个元素之后。用c语言代码解决
以下是用 C 语言实现的代码:
```c
#include<stdio.h>
int main()
{
int n;
while (scanf("%d", &n) != EOF)
{
int a[130][130] = {0};
int i = 1, j = 1, k = 1;
while (k <= n * n)
{
while (i <= n && a[i][j] == 0)
{
a[i][j] = k;
k++;
i++;
}
i--;
j++;
while (j <= n && a[i][j] == 0)
{
a[i][j] = k;
k++;
j++;
}
j--;
i--;
while (i >= 1 && a[i][j] == 0)
{
a[i][j] = k;
k++;
i--;
}
i++;
j--;
while (j >= 1 && a[i][j] == 0)
{
a[i][j] = k;
k++;
j--;
}
j++;
i++;
}
for (int p = 1; p <= n; p++)
{
for (int q = 1; q <= n; q++)
{
printf("%d", a[p][q]);
if (q != n)
{
printf(" ");
}
}
printf("\n");
}
printf("\n");
}
return 0;
}
```
解释一下思路:我们可以用一个二维数组 `a` 来存储蛇形矩阵,初始化为 0。从左上角开始,按照蛇形状的顺序,依次将数字 1 到 n^2 填入二维数组中。在填数字的过程中,我们需要注意边界条件,避免数组越界。最后,按照要求输出蛇形矩阵即可。
c++编码。课程设计内容二 四则运算达式求解(要求分别用中级和后缀两种方法,并设计求解界面) 1.4+2x(--30)-60÷5 2.115-(15+20) ×2+9÷3 3.(900-16×35)÷34-6 4.600-(30-20)+5x2 5.10+12x(34+46)÷32 6.500÷10-(165+35)x3 7.(53+47)x(86-24)÷20 -150 8.9+(45+38-16)x24÷8 9.26x(304-286)+39+3x5 10. 18x2-900÷(120-20×3)11 972÷18+35x(19-10)-30 12.6×58-(175+89)+(8+4)13.(75+49)÷(75-44)+5×7 14.25x(22+576÷32)-7x60 15.5+(736÷16-27)x8-7 16.11+(564-18x24)÷12 17.48×(32-17)+30-(9-3)18.77÷(13-2)x9-4x14 注:可使用随机数自行设计输入表达式!
好的,这是一个关于C++编程的课程设计题目,要求实现对四则运算表达式的求解,包括中缀表达式和后缀表达式两种方法,并设计一个求解界面。在这里,我会给您一些关于实现的思路和建议。
首先,我们需要了解什么是中缀表达式和后缀表达式。中缀表达式是我们平常所见到的表达式,例如“1+2*3”,而后缀表达式则是将运算符放在操作数之后,例如“1 2 3 * +”。后缀表达式求解的过程较为简单,只需遍历一遍表达式,用栈来存储操作数和运算符,遇到运算符则进行计算即可。而中缀表达式求解的过程较为复杂,需要先将中缀表达式转化为后缀表达式,再用后缀表达式的方法进行计算。
接下来,我们需要考虑如何将中缀表达式转化为后缀表达式。这里提供一种常见的方法:使用两个栈,一个存储操作数,一个存储运算符。遍历中缀表达式,遇到操作数则直接输出,遇到运算符则与运算符栈栈顶元素进行比较,如果优先级高于栈顶元素,则入栈,否则将栈顶元素弹出并输出,直到运算符栈为空或者栈顶元素优先级低于当前运算符,然后将当前运算符入栈。最后将运算符栈中的元素依次弹出并输出即可得到后缀表达式。
然后,我们需要考虑如何计算后缀表达式。同样使用一个栈来存储操作数,遍历后缀表达式,遇到操作数则入栈,遇到运算符则弹出栈顶的两个操作数进行计算,并将结果入栈。最终栈中剩下的元素即为计算结果。
最后,我们需要设计一个求解界面,可以使用C++的图形界面库,例如Qt、MFC等。在界面上显示表达式和计算结果,提供按钮或者输入框供用户输入表达式,点击按钮或者回车后进行求解并将结果显示在界面上。
以上就是关于这个编程题目的一些思路和建议,希望能对您有所帮助!
阅读全文
相关推荐
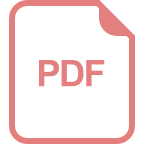
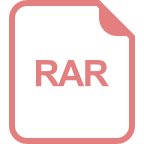
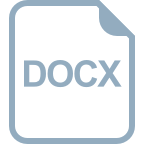
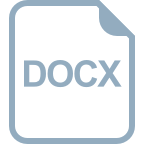
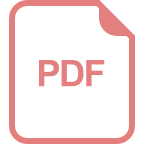
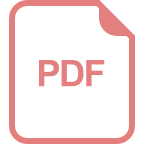








