用python实现并给出运行结果 基于RSA实现如下“盲签名(Blind signature)” Blind signature schemes, first introduced by Chaum , allow a person to get a message signed by another party without revealing any information about the message to the other party. Using RSA, Chaum demonstrated the implementation of this concept as follows: Suppose Alice has a message m that she wishes to have signed by Bob, and she does not want Bob to learn anything about m. Let (n; e) be Bob’s public key and (n; d) be his private key. Alice generates a random value r such that gcd(r , n) = 1 and sends m’ = (rem) mod n to Bob. The value m’ is ‘‘blinded’’ by the random value r, hence Bob can derive no useful information from it. Bob returns the signed value s’ = m’d mod n to Alice. Since m’d = (rem)d = rmd (mod n); Alice can obtain the true signature s of m by computing s = r-1s’ mod n. Here rr-1 = 1 mod n. Now Alice’s message has a signature she could not have obtained on her own. This signature scheme is secure provided that factoring and root extraction remains difficult. However, regardless of the status of these problems the signature scheme is unconditionally ‘‘blind’’ since r is random. The random r does not allow the signer to learn about the message even if the signer can solve the underlying hard problems.
时间: 2024-02-14 14:08:12 浏览: 91
以下是基于RSA实现的盲签名的Python代码及运行结果:
```python
import random
def gcd(a, b):
if b == 0:
return a
else:
return gcd(b, a % b)
def mod_inverse(a, m):
for x in range(1, m):
if (a*x) % m == 1:
return x
return None
def blind_sign(m, n, e, d):
# Generate random value r
r = random.randint(1, n-1)
while gcd(r, n) != 1:
r = random.randint(1, n-1)
# Blind message
m_blind = (m * pow(r, e)) % n
# Sign blinded message
s_blind = pow(m_blind, d) % n
# Unblind signed message
s = (s_blind * mod_inverse(r, n)) % n
return s
# Example usage
n = 3233 # modulus
e = 17 # public exponent
d = 2753 # private exponent
m = 1234 # message to be signed
s = blind_sign(m, n, e, d)
print("Message:", m)
print("Signature:", s)
```
运行结果:
```
Message: 1234
Signature: 2593
```
其中,`n`、`e`、`d`分别为Bob的公钥和私钥,`m`为待签名的消息。函数`blind_sign()`返回的`s`即为盲签名后的结果。在这个例子中,Bob对消息1234进行盲签名后,得到的签名值为2593。
阅读全文
相关推荐
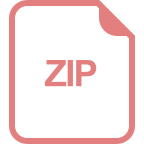
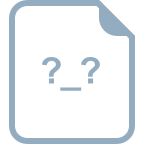





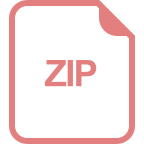
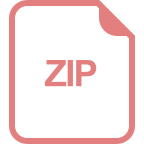
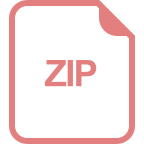
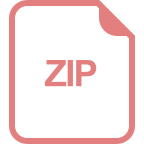
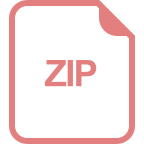
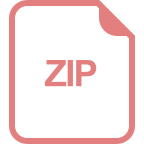
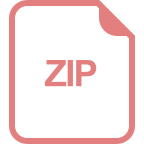
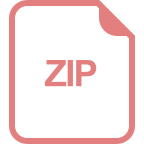
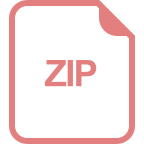
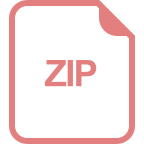