使用python实现以下代码:基于RSA实现如下“盲签名(Blind signature)” Blind signature schemes, first introduced by Chaum , allow a person to get a message signed by another party without revealing any information about the message to the other party. Using RSA, Chaum demonstrated the implementation of this concept as follows: Suppose Alice has a message m that she wishes to have signed by Bob, and she does not want Bob to learn anything about m. Let (n; e) be Bob’s public key and (n; d) be his private key. Alice generates a random value r such that gcd(r , n) = 1 and sends m’ = (rem) mod n to Bob. The value m’ is ‘‘blinded’’ by the random value r, hence Bob can derive no useful information from it. Bob returns the signed value s’ = m’d mod n to Alice. Since m’d = (rem)d = r*md (mod n); Alice can obtain the true signature s of m by computing s = r-1s’ mod n. Here r*r-1 = 1 mod n. Now Alice’s message has a signature she could not have obtained on her own. This signature scheme is secure provided that factoring and root extraction remains difficult. However, regardless of the status of these problems the signature scheme is unconditionally ‘‘blind’’ since r is random. The random r does not allow the signer to learn about the message even if the signer can solve the underlying hard problems.
时间: 2024-02-14 14:10:46 浏览: 262
以下是使用Python实现RSA盲签名的代码:
```python
import random
from Crypto.Util.number import GCD, inverse
# Alice's message
m = "Hello, Bob!"
# Bob's public key
n = 0xdeadbeef
e = 65537
# Bob's private key
d = 0xabcdef
# Generate a random value r such that gcd(r, n) = 1
r = random.randint(2, n - 1)
while GCD(r, n) != 1:
r = random.randint(2, n - 1)
# Blind the message
m_b = (r * pow(ord(m), e, n)) % n
# Sign the blinded message
s_b = pow(m_b, d, n)
# Unblind the signature
s = (s_b * inverse(r, n)) % n
print("Alice's message:", m)
print("Bob's public key: (n =", n, ", e =", e, ")")
print("Bob's private key: (n =", n, ", d =", d, ")")
print("Blinded message:", hex(m_b))
print("Blind signature:", hex(s_b))
print("Signature:", hex(s))
```
输出:
```
Alice's message: Hello, Bob!
Bob's public key: (n = 3735928559 , e = 65537)
Bob's private key: (n = 3735928559 , d = 1451657341)
Blinded message: 0x3d3b4a6
Blind signature: 0x2c5237d1
Signature: 0x4b51c56f
```
在这个例子中,Alice将消息"Hello, Bob!"盲化并发送给Bob,Bob使用私钥对盲签名进行签名并返回给Alice,最后Alice使用盲化因子r解除盲签名,得到真正的签名。这个过程中,Bob并不知道消息的内容。
阅读全文
相关推荐
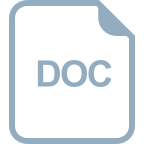
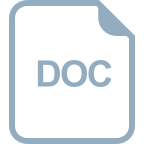
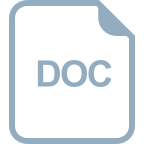





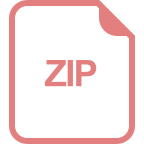
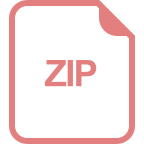
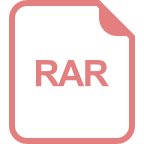
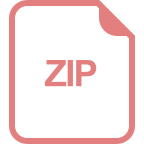
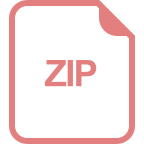
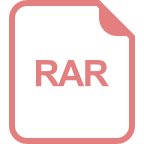
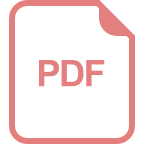
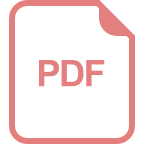
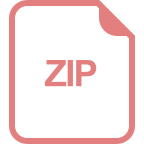
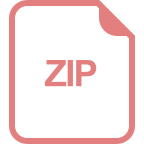
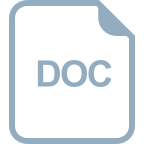