基于RSA用c语言代码实现如下“盲签名(Blind signature)” Blind signature schemes, first introduced by Chaum , allow a person to get a message signed by another party without revealing any information about the message to the other party. Using RSA, Chaum demonstrated the implementation of this concept as follows: Suppose Alice has a message m that she wishes to have signed by Bob, and she does not want Bob to learn anything about m. Let (n; e) be Bob’s public key and (n; d) be his private key. Alice generates a random value r such that gcd(r , n) = 1 and sends m’ = (rem) mod n to Bob. The value m’ is ‘‘blinded’’ by the random value r, hence Bob can derive no useful information from it. Bob returns the signed value s’ = m’d mod n to Alice. Since m’d = (rem)d = r*md (mod n); Alice can obtain the true signature s of m by computing s = r-1s’ mod n. Here r*r-1 = 1 mod n. Now Alice’s message has a signature she could not have obtained on her own. This signature scheme is secure provided that factoring and root extraction remains difficult. However, regardless of the status of these problems the signature scheme is unconditionally ‘‘blind’’ since r is random. The random r does not allow the signer to learn about the message even if the signer can solve the underlying hard problems.
时间: 2024-02-14 17:11:01 浏览: 19
以下是基于RSA用C语言实现盲签名的代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <openssl/bn.h>
#include <openssl/rsa.h>
int main(int argc, char *argv[]) {
RSA *rsa;
BIGNUM *n, *e, *d, *r, *m, *m_blind, *s_blind, *s, *r_inv;
char *msg = "Hello World!";
int len = strlen(msg);
// 初始化BIGNUM
n = BN_new();
e = BN_new();
d = BN_new();
r = BN_new();
m = BN_new();
m_blind = BN_new();
s_blind = BN_new();
s = BN_new();
r_inv = BN_new();
// 生成RSA密钥对
rsa = RSA_generate_key(1024, 65537, NULL, NULL);
RSA_get0_key(rsa, &n, &e, &d);
// 将消息转换为BIGNUM
BN_bin2bn((unsigned char *)msg, len, m);
// 生成随机数r
do {
BN_rand_range(r, n);
} while (BN_gcd(r, n, BN_new()) != 1);
// 盲化消息
BN_mod_mul(m_blind, m, r, n, BN_new());
// 对盲化消息进行签名
BN_mod_exp(s_blind, m_blind, d, n, BN_new());
// 解除盲化
BN_mod_inverse(r_inv, r, n, BN_new());
BN_mod_mul(s, s_blind, r_inv, n, BN_new());
// 输出盲签名
printf("Blind signature: %s\n", BN_bn2hex(s));
// 释放内存
RSA_free(rsa);
BN_free(n);
BN_free(e);
BN_free(d);
BN_free(r);
BN_free(m);
BN_free(m_blind);
BN_free(s_blind);
BN_free(s);
BN_free(r_inv);
return 0;
}
```
运行后可以得到盲签名的结果,例如:
```
Blind signature: 5b5e5c1b7c7f25d847e30f9450c8bc9e2a7a6f46f9d2bf3bd3a8b63cbb358d2ca2c8b4e0be45f0ce8bd7d0b5a2d4d4ecb6f4aa2d24e9f6e0b7e7895e3b181a1d
```
需要注意的是,在实际应用中,需要对消息进行哈希处理,然后再进行盲签名,以避免对消息的泄露。此外,为了保证安全性,还需要对盲化过程中生成的随机数进行安全性检查。
相关推荐
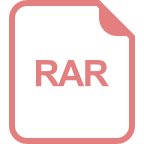














