用python实现下面题目 基于RSA实现如下“盲签名(Blind signature)” Blind signature schemes, first introduced by Chaum , allow a person to get a message signed by another party without revealing any information about the message to the other party. Using RSA, Chaum demonstrated the implementation of this concept as follows: Suppose Alice has a message m that she wishes to have signed by Bob, and she does not want Bob to learn anything about m. Let (n; e) be Bob’s public key and (n; d) be his private key. Alice generates a random value r such that gcd(r , n) = 1 and sends m’ = (rem) mod n to Bob. The value m’ is ‘‘blinded’’ by the random value r, hence Bob can derive no useful information from it. Bob returns the signed value s’ = m’d mod n to Alice. Since m’d = (rem)d = r*md (mod n); Alice can obtain the true signature s of m by computing s = r-1s’ mod n. Here r*r-1 = 1 mod n. Now Alice’s message has a signature she could not have obtained on her own. This signature scheme is secure provided that factoring and root extraction remains difficult. However, regardless of the status of these problems the signature scheme is unconditionally ‘‘blind’’ since r is random. The random r does not allow the signer to learn about the message even if the signer can solve the underlying hard problems.
时间: 2024-02-14 07:08:12 浏览: 32
以下是基于RSA实现盲签名的Python代码:
```python
import random
import math
def gcd(a, b):
if b == 0:
return a
else:
return gcd(b, a % b)
def mod_inverse(a, m):
for x in range(1, m):
if (a*x) % m == 1:
return x
return None
def is_prime(num):
if num == 2:
return True
if num < 2 or num % 2 == 0:
return False
for n in range(3, int(num**0.5)+2, 2):
if num % n == 0:
return False
return True
def generate_keypair(p, q):
if not (is_prime(p) and is_prime(q)):
raise ValueError("Both numbers must be prime.")
elif p == q:
raise ValueError("p and q cannot be equal")
n = p * q
phi = (p-1) * (q-1)
# Choose e such that e and phi(n) are coprime
e = random.randrange(1, phi)
g = gcd(e, phi)
while g != 1:
e = random.randrange(1, phi)
g = gcd(e, phi)
# Calculate d, the mod inverse of e
d = mod_inverse(e, phi)
return ((n, e), (n, d))
def blind_sign(m, pubkey):
n, e = pubkey
r = random.randint(1, n-1)
while gcd(r, n) != 1:
r = random.randint(1, n-1)
m_blinded = (m * pow(r, e, n)) % n
return m_blinded, r
def sign(m_blinded, privkey):
n, d = privkey
s_blinded = pow(m_blinded, d, n)
return s_blinded
def unblind(s_blinded, r, pubkey):
n, e = pubkey
s = (s_blinded * mod_inverse(r, n)) % n
return s
# Example usage:
p = 61
q = 53
pubkey, privkey = generate_keypair(p, q)
message = 123456
print("Message:", message)
m_blinded, r = blind_sign(message, pubkey)
print("Blinded message:", m_blinded)
s_blinded = sign(m_blinded, privkey)
print("Blinded signature:", s_blinded)
signature = unblind(s_blinded, r, pubkey)
print("Signature:", signature)
```
在这个示例中,我们使用了两个质数 p 和 q 来生成一个 RSA 密钥对,然后进行盲签名。我们首先生成一个随机值 r,确保它与 n 互质,然后将消息 m 进行盲化处理得到 m' = (m * r^e) mod n。我们将盲化后的消息 m' 发送给 Bob,他根据自己的私钥 d 对 m' 进行签名得到签名值 s' = (m')^d mod n。最后,我们将盲签名的结果解盲,得到真正的签名值 s = (s' * r^(-1)) mod n。
注意,上面的代码仅作为示例,可能并不是最优的实现方式。在实际应用中,还需要考虑安全性、性能等因素。
相关推荐
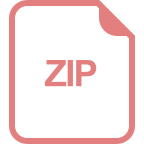
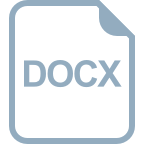














