同时几个线程对串口进行写数据,每个线程等待串口回复数据后,才能进行判断函数。用c语言写出来
时间: 2024-02-03 15:12:22 浏览: 164
以下是一个示例代码,其中使用了 POSIX 线程库中的互斥锁和条件变量来实现线程同步:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <pthread.h>
#include <unistd.h>
#include <fcntl.h>
#include <termios.h>
#define SERIAL_PORT "/dev/ttyUSB0" // 串口设备文件路径
#define BAUD_RATE B9600 // 波特率
char buffer[1024]; // 读写缓冲区
int fd; // 串口文件描述符
pthread_mutex_t mutex; // 互斥锁
pthread_cond_t cond; // 条件变量
int num_threads = 0; // 等待回复的线程数
void* thread_func(void* arg)
{
int thread_id = *(int*)arg;
char request[64];
sprintf(request, "request from thread %d", thread_id);
// 加锁
pthread_mutex_lock(&mutex);
// 发送请求
write(fd, request, strlen(request));
printf("Thread %d sent request: %s\n", thread_id, request);
// 等待回复
num_threads++;
pthread_cond_wait(&cond, &mutex);
num_threads--;
// 解锁
pthread_mutex_unlock(&mutex);
// 判断回复
if (strstr(buffer, request))
{
printf("Thread %d received correct response: %s\n", thread_id, buffer);
}
else
{
printf("Thread %d received incorrect response: %s\n", thread_id, buffer);
}
pthread_exit(NULL);
}
int main()
{
// 打开串口
fd = open(SERIAL_PORT, O_RDWR | O_NOCTTY);
if (fd < 0)
{
perror("open");
exit(1);
}
// 配置串口
struct termios options;
tcgetattr(fd, &options);
cfsetispeed(&options, BAUD_RATE);
cfsetospeed(&options, BAUD_RATE);
options.c_cflag &= ~PARENB;
options.c_cflag &= ~CSTOPB;
options.c_cflag &= ~CSIZE;
options.c_cflag |= CS8;
options.c_cflag &= ~CRTSCTS;
options.c_cflag |= CREAD | CLOCAL;
options.c_iflag &= ~(IXON | IXOFF | IXANY);
options.c_lflag &= ~(ICANON | ECHO | ECHOE | ISIG);
options.c_oflag &= ~OPOST;
options.c_cc[VMIN] = 0;
options.c_cc[VTIME] = 100;
tcsetattr(fd, TCSANOW, &options);
// 初始化互斥锁和条件变量
pthread_mutex_init(&mutex, NULL);
pthread_cond_init(&cond, NULL);
// 创建线程
pthread_t threads[3];
int thread_ids[3] = {1, 2, 3};
for (int i = 0; i < 3; i++)
{
pthread_create(&threads[i], NULL, thread_func, &thread_ids[i]);
}
// 读取回复
while (1)
{
int n = read(fd, buffer, sizeof(buffer));
if (n > 0)
{
// 唤醒等待回复的线程
pthread_mutex_lock(&mutex);
if (num_threads > 0)
{
pthread_cond_signal(&cond);
}
pthread_mutex_unlock(&mutex);
}
}
// 关闭串口
close(fd);
// 销毁互斥锁和条件变量
pthread_mutex_destroy(&mutex);
pthread_cond_destroy(&cond);
return 0;
}
```
这个示例代码中,每个线程都会向串口发送一个请求,并且等待回复。当有回复到达时,会唤醒等待回复的线程。主线程负责不断读取串口数据,如果有数据到达,则会唤醒等待回复的线程。线程间的同步是通过互斥锁和条件变量实现的。
阅读全文
相关推荐
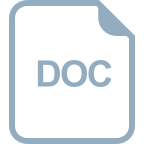
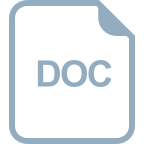
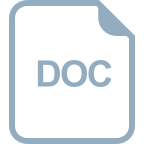




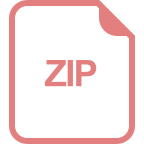
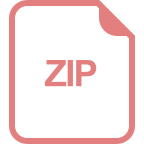
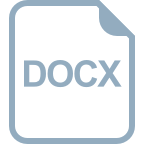
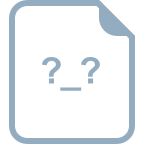
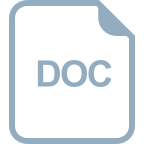
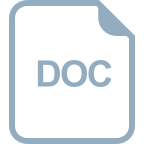
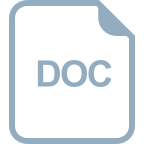
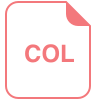



