vs使用MFC模块 Bresenham 画圆算法的完整工程
时间: 2024-02-07 21:01:47 浏览: 37
下面是在 Visual Studio 中使用 MFC 模块和 Bresenham 算法绘制圆形的完整工程:
1. 创建一个空的 MFC 应用程序项目,命名为 `MFCBresenhamCircle`。
2. 在资源编辑器中添加一个 `CPaintDC` 控件,并设置其 ID 为 `IDC_PAINTDC`。
3. 打开 `MainFrm.h` 文件,在 `CMainFrame` 类的 `protected` 声明段中添加以下代码:
```c++
class CMainFrame : public CFrameWnd
{
// ...
protected:
afx_msg void OnPaint();
DECLARE_MESSAGE_MAP()
};
```
4. 在 `MainFrm.cpp` 文件中实现 `OnPaint` 函数和消息映射:
```c++
void CMainFrame::OnPaint()
{
CPaintDC dc(this);
int x0 = dc.GetDeviceCaps(HORZRES) / 2;
int y0 = dc.GetDeviceCaps(VERTRES) / 2;
int r = 100;
CPen pen(PS_SOLID, 2, RGB(255, 255, 255));
CPen* pOldPen = dc.SelectObject(&pen);
int x = r, y = 0;
int decision = 3 - 2 * r;
while (x >= y)
{
// draw the eight octants
dc.SetPixel(x0 + x, y0 + y, RGB(255, 255, 255));
dc.SetPixel(x0 + y, y0 + x, RGB(255, 255, 255));
dc.SetPixel(x0 - y, y0 + x, RGB(255, 255, 255));
dc.SetPixel(x0 - x, y0 + y, RGB(255, 255, 255));
dc.SetPixel(x0 - x, y0 - y, RGB(255, 255, 255));
dc.SetPixel(x0 - y, y0 - x, RGB(255, 255, 255));
dc.SetPixel(x0 + y, y0 - x, RGB(255, 255, 255));
dc.SetPixel(x0 + x, y0 - y, RGB(255, 255, 255));
y++;
if (decision <= 0)
{
decision = decision + 4 * y + 6;
}
else
{
x--;
decision = decision + 4 * (y - x) + 10;
}
}
dc.SelectObject(pOldPen);
}
BEGIN_MESSAGE_MAP(CMainFrame, CFrameWnd)
ON_WM_PAINT()
END_MESSAGE_MAP()
```
5. 打开 `MFCBresenhamCircle.cpp` 文件,在 `InitInstance` 函数中添加以下代码:
```c++
BOOL CMFCBresenhamCircleApp::InitInstance()
{
// ...
CMainFrame* pMainFrame = new CMainFrame;
if (!pMainFrame->LoadFrame(IDR_MAINFRAME))
{
return FALSE;
}
m_pMainWnd = pMainFrame;
pMainFrame->ShowWindow(SW_SHOW);
pMainFrame->UpdateWindow();
return TRUE;
}
```
6. 编译和运行程序,应该会看到一个白色的圆形在窗口中心。
注意:如果你想在 MFC 应用程序中使用 `SetPixel` 函数绘制图形,你需要将 `CPaintDC` 控件添加到视图类中,并在视图类的 `OnDraw` 函数中调用 `SetPixel` 函数。
相关推荐
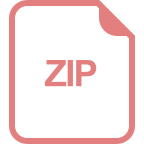
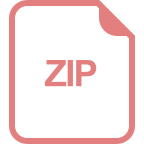














