用java生成一个莲花绽放图
时间: 2023-07-04 14:12:38 浏览: 152
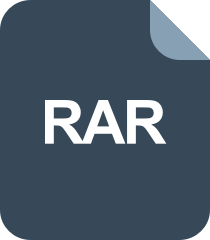
JAVA特效烟花绽放
好的,生成莲花绽放图可以使用Java的Graphics2D类来实现。下面是一个简单的实现:
```java
import java.awt.Color;
import java.awt.Graphics;
import java.awt.Graphics2D;
import java.awt.RenderingHints;
import java.awt.geom.Area;
import java.awt.geom.Ellipse2D;
import java.awt.geom.GeneralPath;
import javax.swing.JFrame;
import javax.swing.JPanel;
public class LotusFlower extends JPanel {
private static final long serialVersionUID = 1L;
private static final int WIDTH = 500;
private static final int HEIGHT = 500;
private double angle = 0.0;
private double size = 0.0;
public LotusFlower() {
JFrame frame = new JFrame();
frame.add(this);
frame.setTitle("莲花绽放图");
frame.setSize(WIDTH, HEIGHT);
frame.setVisible(true);
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
}
public void paintComponent(Graphics g) {
Graphics2D g2d = (Graphics2D) g;
g2d.setRenderingHint(RenderingHints.KEY_ANTIALIASING, RenderingHints.VALUE_ANTIALIAS_ON);
g2d.setBackground(Color.BLACK);
g2d.clearRect(0, 0, WIDTH, HEIGHT);
g2d.translate(WIDTH / 2, HEIGHT / 2);
double r = 0.0;
double x = 0.0;
double y = 0.0;
double startAngle = 0.0;
double endAngle = 0.0;
for (int i = 0; i < 6; i++) {
r = 50.0;
size = 20.0;
for (int j = 0; j < 10; j++) {
x = r * Math.cos(angle);
y = r * Math.sin(angle);
Ellipse2D.Double circle = new Ellipse2D.Double(x - size / 2, y - size / 2, size, size);
g2d.setColor(Color.getHSBColor((float) (angle / (2 * Math.PI)), 1.0f, 1.0f));
g2d.draw(circle);
r += size;
size += 1.5;
}
angle += Math.PI / 3.0;
}
GeneralPath path = new GeneralPath();
path.moveTo(0.0, 0.0);
for (int i = 0; i < 6; i++) {
startAngle = angle - Math.PI / 6.0;
endAngle = angle + Math.PI / 6.0;
x = 150.0 * Math.cos(startAngle);
y = 150.0 * Math.sin(startAngle);
path.quadTo(0.0, 0.0, x, y);
for (double j = startAngle; j <= endAngle; j += 0.01) {
x = 150.0 * Math.cos(j);
y = 150.0 * Math.sin(j);
Ellipse2D.Double circle = new Ellipse2D.Double(x - size / 2, y - size / 2, size, size);
g2d.setColor(Color.getHSBColor((float) (j / (2 * Math.PI)), 1.0f, 1.0f));
g2d.fill(circle);
}
angle += Math.PI / 3.0;
}
path.closePath();
Area area = new Area(path);
g2d.setColor(Color.WHITE);
g2d.fill(area);
}
public static void main(String[] args) {
new LotusFlower();
}
}
```
这个程序会生成一个黑色背景的窗口,在窗口中绘制出一个莲花绽放图。运行程序,你会看到一个不断变化的莲花,最后绽放成一个白色的大花瓣。
阅读全文
相关推荐
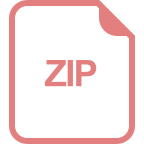

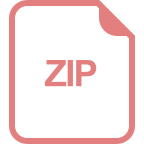
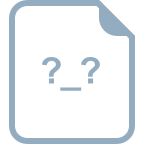
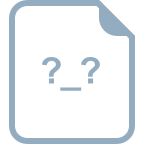
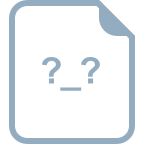
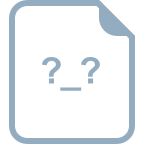
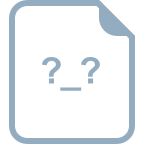
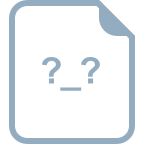
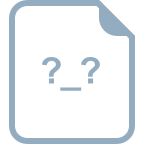
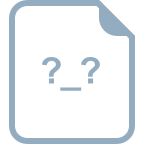
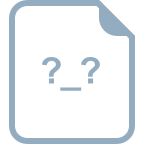
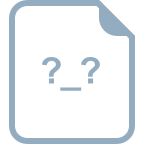
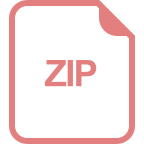
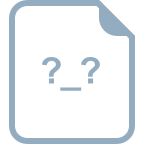
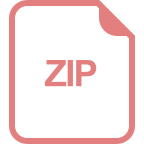
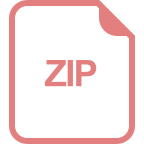