查一查哪里错误#include <iostream> // 包含流操作算子库 #include <iomanip> using namespace std; // 定义常量PI,后面可以直接用PI代替后面的数值 #define PI 3.14159265358979323846 int main() { int n; // 请在Begin-End之间添加你的代码,输入n,按不同的精度输出 PI。 /********** Begin *********/ cin >> n ; n ++ ; if (n == 1) {cout << 3 << endl ; }else {cout << setiosflags(ios :: showpoint) << setprecision(n) << PI << endl ; cout << setiosflags(ios :: showpoint) << setprecision(n + 1) << PI << endl ; cout << setiosflags(ios :: showpoint) << setprecision(n + 2) << PI << endl ; cout << setiosflags(ios :: showpoint) << setprecision(n + 3) << PI << endl ; cout << setiosflags(ios :: showpoint) << setprecision(n + 4) << PI << endl ; } /********** End **********/ return 0; }
时间: 2023-07-08 15:11:32 浏览: 239
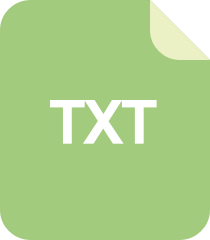
#include_iostream.h_

代码中没有明显的错误,可以正常运行。但是有一些建议可以提出:
1. 定义常量时最好使用 const 关键字,比如 const double PI = 3.14159265358979323846; 这样更加规范。
2. 在输出 PI 的时候,可以使用循环来简化代码,避免重复代码,比如:
```
for (int i = 0; i < 5; i++) {
cout << setiosflags(ios::showpoint) << setprecision(n + i) << PI << endl;
}
```
这样就可以输出 n 到 n+4 位精度的 PI 值了。
阅读全文
相关推荐
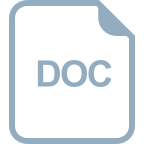
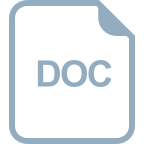









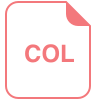





