用 C 语言程序邻接表表示节点的出度值 0 -> 2 -> 1 1 2 -> 3 3 -> 0
时间: 2023-08-07 19:33:04 浏览: 87
这是一个有向图,可以用邻接表来表示。邻接表是一个数组,其中每个元素都指向一个链表,链表中存储该节点指向的所有节点。
下面是用 C 语言程序实现该图的邻接表表示方法,其中定义了一个结构体来表示链表中的节点,节点中存储了指向的节点的编号和下一个节点的指针:
```c
#include <stdio.h>
#include <stdlib.h>
// 链表节点结构体
typedef struct Node {
int dest;
struct Node* next;
} Node;
// 邻接表结构体
typedef struct Graph {
int numVertices;
Node** adjLists;
} Graph;
// 创建新节点
Node* createNode(int dest) {
Node* newNode = (Node*) malloc(sizeof(Node));
newNode->dest = dest;
newNode->next = NULL;
return newNode;
}
// 创建邻接表
Graph* createGraph(int numVertices) {
Graph* graph = (Graph*) malloc(sizeof(Graph));
graph->numVertices = numVertices;
graph->adjLists = (Node**) malloc(numVertices * sizeof(Node*));
int i;
for (i = 0; i < numVertices; i++) {
graph->adjLists[i] = NULL;
}
return graph;
}
// 添加边
void addEdge(Graph* graph, int src, int dest) {
Node* newNode = createNode(dest);
newNode->next = graph->adjLists[src];
graph->adjLists[src] = newNode;
}
// 输出每个节点的出度
void printOutDegree(Graph* graph) {
int i;
for (i = 0; i < graph->numVertices; i++) {
int outDegree = 0;
Node* temp = graph->adjLists[i];
while (temp != NULL) {
outDegree++;
temp = temp->next;
}
printf("Node %d has out degree %d\n", i, outDegree);
}
}
int main() {
Graph* graph = createGraph(4);
addEdge(graph, 0, 2);
addEdge(graph, 2, 1);
addEdge(graph, 2, 3);
addEdge(graph, 3, 0);
printOutDegree(graph);
return 0;
}
```
输出结果为:
```
Node 0 has out degree 1
Node 1 has out degree 0
Node 2 has out degree 2
Node 3 has out degree 1
```
可以看到,节点0的出度为1,节点1的出度为0,节点2的出度为2,节点3的出度为1。
阅读全文
相关推荐
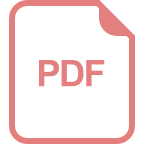





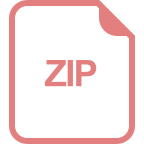








